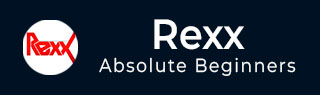
- Rexx Tutorial
- Rexx - Home
- Rexx - Overview
- Rexx - Environment
- Rexx - Installation
- Rexx - Installation of Plugin-Ins
- Rexx - Basic Syntax
- Rexx - Datatypes
- Rexx - Variables
- Rexx - Operators
- Rexx - Arrays
- Rexx - Loops
- Rexx - Decision Making
- Rexx - Numbers
- Rexx - Strings
- Rexx - Functions
- Rexx - Stacks
- Rexx - File I/O
- Rexx - Functions For Files
- Rexx - Subroutines
- Rexx - Built-In Functions
- Rexx - System Commands
- Rexx - XML
- Rexx - Regina
- Rexx - Parsing
- Rexx - Signals
- Rexx - Debugging
- Rexx - Error Handling
- Rexx - Object Oriented
- Rexx - Portability
- Rexx - Extended Functions
- Rexx - Instructions
- Rexx - Implementations
- Rexx - Netrexx
- Rexx - Brexx
- Rexx - Databases
- Handheld & Embedded
- Rexx - Performance
- Rexx - Best Programming Practices
- Rexx - Graphical User Interface
- Rexx - Reginald
- Rexx - Web Programming
- Rexx Useful Resources
- Rexx - Quick Guide
- Rexx - Useful Resources
- Rexx - Discussion
Rexx - Performance
One of the key aspects of any programming language is the performance of the application. Special practices need to be taken care of to ensure that the application’s performance is not hampered. Let’s look at some of the considerations described in steps for better understanding −
Step 1 − Try to reduce the number of instructions – In Rexx each instruction carries an overhead. So try to reduce the number of instructions in your program. An example of instructions that can be redesigned is shown below.
Instead of using multiple if else statements one can use the parse statement. So like in the following program, instead of having an if condition for each value, and getting the value of word1, word2, word3 and word4, use the parse statement.
/* Main program */ parse value 'This is a Tutorial' with word1 word2 word3 word4 say "'"word1"'" say "'"word2"'" say "'"word3"'" say "'"word4"'"
Step 2 − Try to combine multiple statements into one statement. An example is shown below.
Suppose if you had the following code which did the assignment for – a and b and passed it to a method called proc.
do i = 1 to 100 a = 0 b = 1 call proc a,b end
You can easily replace the above given code with the following code using the parse statement.
do i = 1 for 100 parse value 0 1 with a, b, call proc a,b end
Step 3 − Try to replace the do..to loop with the do..for loop wherever possible. This is normally recommended when the control variable is being iterated through a loop.
/* Main program */ do i = 1 to 10 say i end
The above program should be replaced by the following program.
/* Main program */ do i = 1 for 10 say i end
Step 4 − If possible, remove the for condition from a do loop as shown in the following program. If the control variable is not required, then just put the end value in the do loop as shown below.
/* Main program */ do 10 say hello end
Step 5 − In a select clause, whatever u feel is the best condition which will be evaluated needs to put first in the when clause. So in the following example, if we know that 1 is the most frequent option, we put the when 1 clause as the first clause in the select statement.
/* Main program */ select when 1 then say'1' when 2 then say'2' otherwise say '3' end