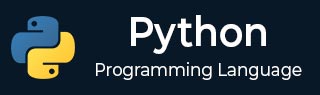
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
The zipfile Module
ZIP is one of the most popular file formats used for archiving and compression. It has been in use since the days of MSDOS and PC and has been used by famous PKZIP application.
Python's standard library provides zipfile module with classes that facilitate the tools for creating, extracting, reading and writing to ZIP archives.
ZipFile() function
This function returns a ZipFile object from a file parameter which can be a string or file object as created by built-in open() function. The function needs a mode parameter whose default value is 'r' although it can take 'w' or 'a' value for opening the archive in read, write or append mode respectively.
The archive by default is uncompressed. To specify the type of compression algorithm to be used, one of the constants has to be assigned to compression parameter.
zipfile.ZIP_STORED |
for an uncompressed archive member. |
zipfile.ZIP_DEFLATED |
for the usual ZIP compression method. This requires the zlib module. |
zipfile.ZIP_BZIP2 |
for the BZIP2 compression method. This requires the bz2 module. |
zipfile.ZIP_LZMA |
for the LZMA compression method. This requires the lzma module. |
The ZipFile object uses following methods −
write() method
This method adds the given file to the ZipFile object.
import zipfile newzip=zipfile.ZipFile('newzip.zip','w') newzip.write('zen.txt') newzip.close()
This creates newzip.zip file in th current directory. Additional file can be added to already existing archive by opening it in append mode ('a' as the mode).
import zipfile newzip=zipfile.ZipFile('newzip.zip','a') newzip.write('json.txt') newzip.close()
read() method
This method reads data from a particular file in the archive.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') data = newzip.read('json.txt') print (data) newzip.close()
Output
b'["Rakesh", {"marks": [50, 60, 70]}]'
printdir() method
This method lists all file in given archive.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') newzip.printdir() newzip.close()
Output
File Name Modified Size zen.txt 2023-03-30 21:55:48 132 json.txt 2023-04-03 22:01:56 35
extract() method
This method extracts a specified file from archive by default to current directory or to one given as second parameter to it.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') newzip.extract('json.txt', 'newdir') newzip.close()
extractall() method
This method extracts all files in the archive to current directory by default. Specify alternate directory if required as parameter.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') newzip.extractall('newdir') newzip.close()
getinfo() method
This method returns ZipInfo object corresponding to the given file. The ZipInfo object contains different metadata information of the file.
Following code obtains ZipInfo object of 'zen.txt' from the archive and retrieves filename, size and date-time information from it.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') info = newzip.getinfo('zen.txt') print (info.filename, info.file_size, info.date_time) newzip.close()
Output
zen.txt 132 (2023, 3, 30, 21, 55, 48)
infolist() method
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') info = newzip.infolist() print (info) newzip.close()
Output
[<ZipInfo filename='zen.txt' filemode='-rw-rw-rw-' file_size=132>, <ZipInfo filename='json.txt' filemode='-rw-rw-rw-' file_size=35>]
namelist() method
This method of ZipFile object returns a list of all files in the archive.
import zipfile newzip=zipfile.ZipFile('newzip.zip','r') info = newzip.namelist() print (info) newzip.close()
Output
['zen.txt', 'json.txt']
setpassword() method
This method sets password parameter which must be provided at the time of extracting the archive.