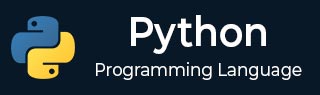
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Theoretic and Representation Functions
ceil() function
The ceil() function from math module returns the ceiling value of x i.e. the smallest integer not less than x.
Syntax
Following is the syntax for the ceil() function −
import math math.ceil(x)
Note − This function is not accessible directly, so we need to import math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This function returns the smallest integer not less than "x".
Example
The following example shows the usage of the ceil() function.
from math import ceil, pi a = -45.17 ceil_a = ceil(a) print ("a: ",a, "ceil(a): ", ceil_a) a = 100.12 ceil_a = ceil(a) print ("a: ",a, "ceil(a): ", ceil_a) a = 100.72 ceil_a = ceil(a) print ("a: ",a, "ceil(a): ", ceil_a) a = pi ceil_a = ceil(a) print ("a: ",a, "ceil(a): ", ceil_a)
When we run the above program, it produces the following output −
a: -45.17 ceil(a): -45 a: 100.12 ceil(a): 101 a: 100.72 ceil(a): 101 a: 3.141592653589793 ceil(a): 4
comb() function
The comb() function in math module returns the number of ways to choose "x" items from "y" items without repetition and without order. It evaluates to n! / (x! * (x-y)!) when x <= y and evaluates to zero when x > y.
Syntax
Following is the syntax for the comb() function −
math.comb(x, y)
Parameters
x − Required. Positive integers of items to choose from.
y − Required. Positive integers of items to choose.
Return value
If the value of "x" is greater than the value of y, it will return 0. If x and y are negative, a ValueError occurs. If both the parameters are not integers, TypeError is raised.
Example
from math import comb x=7 y=5 combinations = comb(x,y) print ("x: ",x, "y: ", y, "combinations: ", combinations) x=5 y=7 combinations = comb(x,y) print ("x: ",x, "y: ", y, "combinations: ", combinations)
It will produce the following output −
x: 7 y: 5 combinations: 21 x: 5 y: 7 combinations: 0
copysign() Function
The copy() function in math module returns Return a float with the magnitude (absolute value) of x but the sign of y.
Syntax
Following is the syntax for the copysign() function −
math.copysign(x, y)
Parameters
- x - a number
- y - a number
Return value
Returns a float with the magnitude (absolute value) of x, but the sign of y.
Example
from math import copysign x=10 y=-20 result = copysign(x,y) print ("x: ",x, "y: ", y, "copysign: ", result) x=-10 y=20 result = copysign(x,y) print ("x: ",x, "y: ", y, "copysign: ", result) x=-10 y= -0.0 result = copysign(x,y) print ("x: ",x, "y: ", y, "copysign: ", result)
It will produce the following output −
x: 10 y: -20 copysign: -10.0 x: -10 y: 20 copysign: 10.0 x: -10 y: -0.0 copysign: -10.0
fabs() Function
The fabs() function in math module returns absolute value of the given number. It always returns a float, even if the parameter is integer.
Syntax
Following is the syntax for the fabs() function −
math.fabs(x)
Parameters
x − a number
Return value
Returns a float with the magnitude (absolute value) of x
Example
from math import fabs x=10.25 result = fabs(x) print ("x: ",x, "fabs value: ", result) x=20 result = fabs(x) print ("x: ",x,"fabs value: ", result) x=-10 result = fabs(x) print ("x: ",x, "fabs value: ", result)
It will produce the following output −
x: 10.25 fabs value: 10.25 x: 20 fabs value: 20.0 x: -10 fabs value: 10.0
factorial() Function
The factorial() function in math module returns the factorial value of the given integer. Factorial of a number is the product of all integers from 1 to that number. Denoted as x! where 4! = 4X3X2X1.
Syntax
Following is the syntax for the factorial() function −
math.factorial(x)
Parameters
x − an integer
Return Value
Returns an integer with product of integers from 1 to x. For negative x, ValueError is raised.
Example
from math import factorial x=10 result = factorial(x) print ("x: ",x, "x! value: ", result) x=5 result = factorial(x) print ("x: ",x,"x! value: ", result) x=-5 result = factorial(x) print ("x: ",x, "x! value: ", result)
It will produce the following output −
x: 10 x! value: 3628800 x: 5 x! value: 120 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 14, in <module> result = factorial(x) ^^^^^^^^^^^^ ValueError: factorial() not defined for negative values
floor() function
The floor() function returns the floor of x, i.e. the largest integer not greater than x.
Syntax
Following is the syntax for the floor() function −
import math math.floor(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This function returns the largest integer not greater than x.
Example
The following example shows the usage of the floor() function.
from math import floor, pi a = -45.17 floor_a = floor(a) print ("a: ",a, "floor(a): ", floor_a) a = 100.12 floor_a = floor(a) print ("a: ",a, "floor(a): ", floor_a) a = 100.72 floor_a = floor(a) print ("a: ",a, "floor(a): ", floor_a) a = pi floor_a = floor(a) print ("a: ",a, "floor(a): ", floor_a)
When we run the above program, it produces the following output −
a: -45.17 floor(a): -46 a: 100.12 floor(a): 100 a: 100.72 floor(a): 100 a: 3.141592653589793 floor(a): 3
fmod() Function
The fmod() function in math module returns same result as x%y. However fmod() gives more accurate result of modulo division than modulo operator.
Syntax
Following is the syntax for the fmod() function −
import math math.fmod(x, y)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Positive or negative number to be divided.
y − Positive or negative number to be divided by.
Return Value
This function returns remainder of x divided by y.
Example
The following example shows the usage of the fmod() function.
from math import fmod a=10 b=2 c=fmod(a,b) print ("a=",a, "b=",b, "fmod(a,b)=", c) a=10 b=4 c=fmod(a,b) print ("a=",a, "b=",b, "fmod(a,b)=", c) a=0 b=10 c=fmod(a,b) print ("a=",a, "b=",b, "fmod(a,b)=", c) a=10 b=1.5 c=fmod(a,b) print ("a=",a, "b=",b, "fmod(a,b)=", c)
When we run the above program, it produces the following output −
a= 10 b= 2 fmod(a,b)= 0.0 a= 10 b= 4 fmod(a,b)= 2.0 a= 0 b= 10 fmod(a,b)= 0.0 a= 10 b= 1.5 fmod(a,b)= 1.0
frexp() Function
The frexp() function in math module returns the mantissa and exponent of x as the pair (m, e). m is a float and e is an integer such that x == m * 2**e exactly.
Syntax
Following is the syntax for the frexp() function −
import math math.frexp(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Positive or negative number.
Return Value
This function returns mantissa and exponent (m,n) such that m*2**e is equal to x.
Example
The following example shows the usage of the frexp() function.
from math import frexp x = 8 y = frexp(x) print ("x:", x, "frex(x):",y) print ("Cross-check") m,n=y x = m*2**n print ("frexp(x): ",y, "x:", x)
It will produce the following output −
x: 8 frex(x): (0.5, 4) Cross-check frexp(x): (0.5, 4) x: 8.0
fsum() Function
The fsum() function in math module returns the floating point sum of all numeric items in an iterable i.e. list, tuple, array.
Syntax
Following is the syntax for the fsum() function −
import math math.sum(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Itrable consisting of numbers. Other types result in TypeError.
Return Value
This function returns sum of all items in the iterable in float.
Example
The following example shows the usage of the fsum() function −
from math import fsum x = [1,2,3,4,5] y = fsum(x) print ("x:", x, "fsum(x):",y) x = (10,11,12) y = fsum(x) print ("x:", x, "fsum(x):",y) x = [1,'a',2] y = fsum(x) print ("x:", x, "fsum(x):",y)
It will produce the following output −
x: [1, 2, 3, 4, 5] fsum(x): 15.0 x: (10, 11, 12) fsum(x): 33.0 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 13, in <module> y = fsum(x) ^^^^^^^ TypeError: must be real number, not str
gcd() function
The gcd() function in math module returns the greatest common divisor of all integer numbers. The returned value is the largest positive integer that is a divisor of all arguments. If all numbers are zero, it returns 0. gcd() with no arguments returns 0.
Syntax
Following is the syntax for the gcd() function −
import math math.gcd(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
- x1, x2 − integers
Return Value
This function greatest common divisor, as integer
Example
The following example shows the usage of the gcd() function −
from math import gcd x, y, z = 12, 8, 24 result = gcd(x, y, z) print ("x: {} y: {} z: {}".format(x,y,z), "gcd(x,y, z):",result) x, y, z = 12, 6, 9 result = gcd(x, y, z) print ("x: {} y: {} z: {}".format(x,y,z), "gcd(x,y, z):",result) x, y = 7, 12 result = gcd(x, y) print ("x: {} y: {}".format(x,y), "gcd(x,y):",result) x, y = 0, 12 result = gcd(x, y) print ("x: {} y: {}".format(x,y), "gcd(x,y):",result)
It will produce the following output −
x: 12 y: 8 z: 24 gcd(x,y, z): 4 x: 12 y: 6 z: 9 gcd(x,y, z): 3 x: 7 y: 12 gcd(x,y): 1 x: 0 y: 12 gcd(x,y): 12
isclose() Function
The isclose() function in math module returns the True if the values of two numeric arguments are close to each other, false otherwise.
Syntax
Following is the syntax for the isclose() function −
import math math.isclose(x,y)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object. The closeness is determined according to given absolute and relative tolerances.
Parameters
x − The first value to check for closeness.
y − The second value to check for closeness.
rel_tol − The relative tolerance (optional).
abs_tol − The minimum absolute tolerance. (optional).
Return Value
This function returns True if x and y are close to each other, False otherwise.
Example
The following example shows the usage of the isclose() function.
from math import isclose x = 2.598 y = 2.597 result = isclose(x, y) print ("x: {} y:{}".format(x,y), "isclose(x,y):",result) x = 5.263 y = 5.263000001 result = isclose(x, y) print ("x: {} y:{}".format(x,y), "isclose(x,y):",result)
It will produce the following output −
x: 2.598 y:2.597 isclose(x,y): False x: 5.263 y:5.263000001 isclose(x,y): True
isfinite() Function
The isfinite() function in math module returns the True if the argument is neither an infinity nor a NaN, and False otherwise.
Syntax
Following is the syntax for the isfinite() function −
import math math.isfinite(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Numeric operand.
Return Value
This function returns True if x is neither infinite nor NaN, False otherwise.
Example
The following example shows the usage of the isfinite() function −
from math import isfinite x = 1.23E-5 result = isfinite(x) print ("x:", x, "isfinite(x):",result) x = 0 result = isfinite(x) print ("x:", x, "isfinite(x):",result) x = float("Inf") result = isfinite(x) print ("x:", x, "isfinite(x):",result)
It will produce the following output −
x: 1.23e-05 isfinite(x): True x: 0 isfinite(x): True x: inf isfinite(x): False
isinf() Function
The isinf() function in math module returns the True if the argument is a positive or negative infinity and False otherwise. It is opposite of isfinite() function.
Syntax
Following is the syntax for the isinf() function −
import math math.isinf(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Numeric operand
Return Value
This function returns True if x is positive or negative infinity, False otherwise.
Example
The following example shows the usage of the isinf() function −
from math import isinf x = 1.23E-5 result = isinf(x) print ("x:", x, "isinf(x):",result) x = float("-Infinity") result = isinf(x) print ("x:", x, "isinf(x):",result) x = float("Inf") result = isinf(x) print ("x:", x, "isinf(x):",result)
It will produce the following output −
x: 1.23e-05 isinf(x): False x: -inf isinf(x): True x: inf isinf(x): True
isnan() Function
The isnan() function in math module returns the True if x is a NaN (not a number), and False otherwise.
Syntax
Following is the syntax for the isnan() function −
import math math.isnan(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Numeric operand
Return Value
This function returns Return True if x is a NaN (not a number), and False otherwise.
Example
The following example shows the usage of the isnan() function −
from math import isnan x = 156.78 result = isnan(x) print ("x:", x, "isnan(x):",result) x = float("-Infinity") result = isnan(x) print ("x:", x, "isnan(x):",result) x = float("NaN") result = isnan(x) print ("x:", x, "isnan(x):",result) x = "NaN" result = isnan(x) print ("x:", x, "isnan(x):",result)
It will produce the following output −
x: 156.78 isnan(x): False x: -inf isnan(x): False x: nan isnan(x): True Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 16, in <module> result = isnan(x) ^^^^^^^^ TypeError: must be real number, not str
Note that "NaN" is a string and not NaN numeric operand.
isqrt() Function
The isqrt() function in math module returns Return the integer square root of the nonnegative integer. This is the floor of the exact square root of given positive integer. The Square of the resultant value is less than or equal to the argument number.
Syntax
Following is the syntax for the isqrt() function −
import math math.isqrt(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Non-negative integer.
Return Value
This function returns Return square root number rounded downwards to the nearest integer.
Example
The following example shows the usage of the isqrt() function −
from math import isqrt, sqrt x = 12 y = isqrt(x) z = sqrt(x) print ("x:", x, "isqrt(x):",y, "sqrt(x):", z) x = 16 y = isqrt(x) z = sqrt(x) print ("x:", x, "isqrt(x):",y, "sqrt(x):", z) x = -100 y = isqrt(x) z = sqrt(x) print ("x:", x, "isqrt(x):",y, "sqrt(x):", z)
For comparison, value of sqrt() function is also computed. sqrt() returns float, isqrt() returns integer. For isqrt(), the number must be non-negative.
It will produce the following output −
x: 12 isqrt(x): 3 sqrt(x): 3.4641016151377544 x: 16 isqrt(x): 4 sqrt(x): 4.0 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 14, in <module> y = isqrt(x) ^^^^^^^^ ValueError: isqrt() argument must be nonnegative
lcm() Function
The lcm() function in math module returns Return least common multiple of two or more integer arguments. For nonzero arguments, the function returns the smallest positive integer that is a multiple of all arguments. If any of the arguments is zero, then the returned value is 0.
Syntax
Following is the syntax for the lcm() function −
import math math.lcm(x1, x2, . . )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x1, x2, . . − Integer
Return Value
This function returns integer that is least common multiple of all integers.
Example
The following example shows the usage of the lcm() function −
from math import lcm x = 4 y = 12 z = 9 result = lcm(x,y,z) print ("x: {} y: {} z: {}".format(x,y,z), "lcm(x,y,z):", result) x = 5 y = 15 z = 0 result = lcm(x,y,z) print ("x: {} y: {} z: {}".format(x,y,z), "lcm(x,y,z):", result) x = 4 y = 3 z = 6 result = lcm(x,y,z) print ("x: {} y: {} z: {}".format(x,y,z), "lcm(x,y,z):", result)
It will produce the following output −
x: 4 y: 12 z: 9 lcm(x,y,z): 36 x: 5 y: 15 z: 0 lcm(x,y,z): 0 x: 4 y: 3 z: 6 lcm(x,y,z): 12
ldexp() Function
The ldexp() function in math module returns product of first number with exponent of second number. So, ldexp(x,y) returns x*2**y. This is inverse of frexp() function.
Syntax
Following is the syntax for the ldexp() function −
import math math.lcm(x,y)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Positive or negative number.
y − Positive or negative number.
Return Value
This function returns x*2**y
Example
The following example shows the usage of the ldexp() function −
from math import ldexp, frexp x = 0.5 y = 4 z = ldexp(x,y) print ("x: {} y: {}".format(x,y), "ldexp(x,y)", z) print ("Cross-check") x,y = frexp(z) print ("ldexp value:", z, "frexp(z):",x,y )
It will produce the following output &minu;
x: 0.5 y: 4 ldexp(x,y) 8.0 Cross-check ldexp value: 8.0 frexp(z): 0.5 4
modf() Function
The modf() method returns the fractional and integer parts of x in a two-item tuple. Both parts have the same sign as x. The integer part is returned as a float.
Syntax
Following is the syntax for the modf() method −
import math math.modf( x )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This method returns the fractional and integer parts of x in a two-item tuple. Both the parts have the same sign as x. The integer part is returned as a float.
Example
The following example shows the usage of the modf() method −
from math import modf, pi a = 100.72 modf_a = modf(a) print ("a: ",a, "modf(a): ", modf_a) a = 19 modf_a = modf(a) print ("a: ",a, "modf(a): ", modf_a) a = pi modf_a = modf(a) print ("a: ",a, "modf(a): ", modf_a)
It will produce the following output −
a: 100.72 modf(a): (0.7199999999999989, 100.0) a: 19 modf(a): (0.0, 19.0) a: 3.141592653589793 modf(a): (0.14159265358979312, 3.0)
nextafter() Function
The nextafter() function returns the next floating-point value after x towards y.
If y>x, x increments.
If y<x, x decrements.
Syntax
Following is the syntax for the nextafter() function −
import math math.nextafter( x, y )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x and y - numeric operands.
Return Value
This function returns the next floating point value.
Example
The following example shows the usage of the nextafter() function −
from math import nextafter x=1.5 y=100 z=nextafter(x, y) print ("x: {} y: {}".format(x,y), "nextafter(x,y)", z) x=1.5 y=float("-inf") z=nextafter(x, y) print ("x: {} y: {}".format(x,y), "nextafter(x,y)", z) x=0 y=float("inf") z=nextafter(x, y) print ("x: {} y: {}".format(x,y), "nextafter(x,y)", z)
It will produce the following output −
x: 1.5 y: 100 nextafter(x,y) 1.5000000000000002 x: 1.5 y: -inf nextafter(x,y) 1.4999999999999998 x: 0 y: inf nextafter(x,y) 5e-324
perm() Function
The perm() function computes the permutation. It returns the number of ways to choose x items from y items without repetition and with order.
Permutation is defined as xPy = x! / (x-y)! when y<=x and evaluates to zero when y>x.
Syntax
Following is the syntax for the perm() function −
import math math.perm( x, y )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Required. Positive integers of items to choose from.
y − Required. Positive integers of items to choose.
Return Value
This function returns the permutation value of xPy = = x! / (x-y)!
Example
The following example shows the usage of the perm() function.
from math import perm x=5 y=3 permutations = perm(x,y) print ("x: ",x, "y: ", y, "permutations: ", permutations) x=3 y=5 permutations = perm(x,y) print ("x: ",x, "y: ", y, "permutations: ", permutations)
It will produce the following output −
x: 5 y: 3 permutations: 60 x: 3 y: 5 permutations: 0
prod() Function
The prod() function computes product of all numeric items in the iterable (list, tuple) given as argument. Second argument is start with default value 1.
Syntax
Following is the syntax for the prod() function −
import math math.prod(iterable, start)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
iterable − Required. Must contain numeric operands.
start − Default is 1.
Return Value
This function returns product of all items in the iterable.
Example
The following example shows the usage of the prod() function −
from math import prod x = [2,3,4] product = prod(x) print ("x: ",x, "product: ", product) x = (5,10,15) product = prod(x) print ("x: ",x, "product: ", product) x = [2,3,4] y = 3 product = prod(x, start=y) print ("x: ",x,"start:", y, "product: ", product)
It will produce the following output −
x: [2, 3, 4] product: 24 x: (5, 10, 15) product: 750 x: [2, 3, 4] start: 3 product: 72
remainder() Function
The remainder() function returns the remainder of x with respect to y. This is the difference x - n*y, where n is the integer closest to the quotient x / y. If x / y is exactly halfway between two consecutive integers, the nearest even integer is used for n.
Syntax
Following is the syntax for the remainder() function −
import math math.remainder(x, y)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object
Parameters
x, y: numeric operands. Y must be non-zero.
Return Value
This function returns the remainder of x/y.
Example
The following example shows the usage of the remainder() function −
from math import remainder x = 18 y= 4 rem = remainder(x, y) print ("x: ",x, "y:", y, "remainder: ", rem) x = 22 y= 4 rem = remainder(x, y) print ("x: ",x, "y:", y, "remainder: ", rem) x = 15 y= float("inf") rem = remainder(x, y) print ("x: ",x, "y:", y, "remainder: ", rem) x = 15 y= 0 rem = remainder(x, y) print ("x: ",x, "y:", y, "remainder: ", rem)
It will produce the following output −
x: 18 y: 4 remainder: 2.0 x: 22 y: 4 remainder: -2.0 x: 15 y: inf remainder: 15.0 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 20, in <module> rem = remainder(x, y) ^^^^^^^^^^^^^^^ ValueError: math domain error
trunc() Function
The trunc() function returns integral part of the number, removing the fractional part. trunc() is equivalent to floor() for positive x, and equivalent to ceil() for negative x.
Syntax
Following is the syntax for the trunc() function −
import math math.trunc(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Numeric operands
Return Value
This function returns integer part of the operand.
Example
The following example shows the usage of the trunc() function −
from math import trunc x = 5.79 rem = trunc(x) print ("x: ",x, "truncated: ", rem) x = 12.04 rem = trunc(x) print ("x: ",x, "truncated: ", rem) x = -19.98 rem = trunc(x) print ("x: ",x, "truncated: ", rem) x = 15 rem = trunc(x) print ("x: ",x, "truncated: ", rem)
It will produce the following output −
x: 5.79 truncated: 5 x: 12.04 truncated: 12 x: -19.98 truncated: -19 x: 15 truncated: 15
ulp() Function
ULP stands for "Unit in the Last Place". The ulp() function Return the value of the least significant bit of the float x. trunc() is equivalent to floor() for positive x, and equivalent to ceil() for negative x.
Different scenarios −
If x is a NaN, return x.
If x is negative, return ulp(-x).
If x is a positive infinity, return x.
If x is equal to zero, return the smallest positive denormalized representable float.
If x is equal to the largest positive representable float, return the value of the least significant bit of x.
If x is a positive finite number, return the value of the least significant bit of x, such that the first float bigger than x is x + ulp(x).
Syntax
Following is the syntax for the ulp() function −
import math math.ulp(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − Numeric operands
Return Value
This function least significant bit od float x.
Example
The following example shows the usage of the ulp() function −
from math import ulp x = float('nan') rem = ulp(x) print ("x: ",x, "ULP: ", rem) x = 0.0 rem = ulp(x) print ("x: ",x, "ULP: ", rem) x = -10 rem = ulp(x) print ("x: ",x, "ULP: ", rem) x = 15 rem = ulp(x) print ("x: ",x, "ULP: ", rem)
It will produce the following output −
x: nan ULP: nan x: 0.0 ULP: 5e-324 x: -10 ULP: 1.7763568394002505e-15 x: 15 ULP: 1.7763568394002505e-15