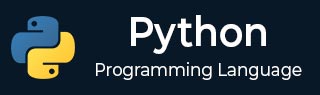
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python math.e Constant
The Python math.e represents the mathematical constant e, which is approximately equal to 2.71828. It is a predefined value available in Python's math module and is commonly used in mathematical calculations involving exponential growth and decay, such as compound interest, population growth, and various scientific and engineering problems.
In general mathematics, e is a special number known as Euler's number, named after the Swiss mathematician Leonhard Euler. It is an irrational number, which means its decimal representation goes on infinitely without repeating.
The constant e is the base of the natural logarithm and is frequently used in mathematical computations. It represents the limit of (1 + 1/n)n as n approaches infinity.
Syntax
Following is the basic syntax of the Python math.e constant −
math.e
Return Value
The constant returns the value of mathematical constant e.
Example 1
In the following example, we are using the math.e constant to calculate the compound interest issued on a principal amount over a specified period of time, given an interest rate. This involves applying the formula for compound interest with continuous compounding, where the base of Euler's number (e) is raised to the power of the interest rate multiplied by time −
import math principal = 1000 rate = 0.05 time = 5 compound_interest = principal * math.e ** (rate * time) print("The compound interest after", time, "years is:", compound_interest)
Output
Following is the output of the above code −
The compound interest after 5 years is: 1284.0254166877414
Example 2
Here, we calculate exponential growth of a quantity over time using Euler's number (e). This is done by computing the final amount after a specified period of time, providing the initial amount, growth rate, and time, using the formula for exponential growth −
import math initial_amount = 10 growth_rate = 0.1 time = 3 final_amount = initial_amount * math.e ** (growth_rate * time) print("The final amount after", time, "years of exponential growth is:", final_amount)
Output
The output obtained is as follows −
The final amount after 3 years of exponential growth is: 13.498588075760033
Example 3
In this example, we approximate the factorial of a number using Stirling's approximation. This involves raising Euler's number (e) to the power of the natural logarithm of the factorial of the number plus one −
import math n = 5 factorial_approximation = math.e ** (math.lgamma(n + 1)) print("The approximation of", n, "! using Stirling's approximation is:", factorial_approximation)
Output
The result produced is as follows −
The approximation of 5 ! using Stirling's approximation is: 120.00000000000006
Example 4
Now, we are calculating the probability density at a specified point "x" for a standard normal distribution using Euler's number (e) −
import math x = 2 mu = 0 sigma = 1 probability_density = (1 / (math.sqrt(2 * math.pi) * sigma)) * math.e ** (-0.5 * ((x - mu) / sigma) ** 2) print("The probability density at x =", x, "for a standard normal distribution is:", probability_density)
Output
We get the output as shown below −
The probability density at x = 2 for a standard normal distribution is: 0.05399096651318806