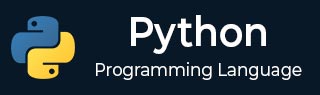
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Power and Logarithmic Functions
Cbrt() Function
The cbrt() function in math module returns the cube root of a number.
Syntax
math.cbrt(x)
Parameters
x − Numeric operand
Return value
The cbrt() function returns the cube root of the given number.
Example
from math import cbrt x = 27 cbr = cbrt(x) print ("x: ",x, "cbrt(x): ", cbr) x = 100 cbr = cbrt(x) print ("x: ",x, "cbrt(x): ", cbr) x = 8.8 cbr = cbrt(x) print ("x: ",x, "cbrt(x): ", cbr)
It will produce the following output −
x: 27 cbrt(x): 3.0 x: 100 cbrt(x): 4.641588833612779 x: 8.8 cbrt(x): 2.0645602309127344
exp() Function
The exp() function returns exponential of x: ex.
Syntax
Following is the syntax for the exp() function −
import math math.exp(x)
Note − This function is not accessible directly. Therefore, we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This method returns exponential of x: ex.
Example
The following example shows the usage of exp() method.
import math # This will import math module print ("math.exp(-45.17) : ", math.exp(-45.17)) print ("math.exp(100.12) : ", math.exp(100.12)) print ("math.exp(100.72) : ", math.exp(100.72)) print ("math.exp(math.pi) : ", math.exp(math.pi))
When we run the above program, it produces the following output −
math.exp(-45.17) : 2.4150062132629406e-20 math.exp(100.12) : 3.0308436140742566e+43 math.exp(100.72) : 5.522557130248187e+43 math.exp(math.pi) : 23.140692632779267
exp2() Function
The exp2() function in math module returns 2 raised to power x. It is equivalent to 2**x.
Syntax
math.exp2(x)
Parameters
x − Numeric operand
Return Value
The function returns 2 raised to x.
Example
from math import exp2 x = 6 val = exp2(x) print ("x: ",x, "exp2(x): ", val) print ("cross-check:", 2**6) x = -3 val = exp2(x) print ("x: ",x, "exp2(x): ", val) x = 2.5 val = exp2(x) print ("x: ",x, "exp2(x): ", val)
It will produce the following output −
x: 6 exp2(x): 64.0 cross-check: 64 x: -3 exp2(x): 0.125 x: 2.5 exp2(x): 5.656854249492381
expm1() Function
The expm1() function in math module computes and returns e raised to the power x, minus 1. Here e is the base of natural logarithms. The expm1() function provides a way to compute this quantity to full precision.
Syntax
math.expm1(x)
Parameters
x − int or float operand.
Return Value
This function return the exponential value of a number − 1.
Example
from math import expm1 x = 6 val = expm1(x) print ("x: ",x, "expm1(x): ", val) x = -3 val = expm1(x) print ("x: ",x, "expm1(x): ", val) x = 2.5 val = expm1(x) print ("x: ",x, "expm1(x): ", val)
It will produce the following output −
x: 6 expm1(x): 402.4287934927351 x: -3 expm1(x): -0.950212931632136 x: 2.5 expm1(x): 11.182493960703473
log() Function
The log() function returns the natural logarithm of x, for x > 0.
Syntax
Following is the syntax for the log() function −
import math math.log( x )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This function returns natural logarithm of x, for x > 0.
Example
The following example shows the usage of the log() method −
import math # This will import math module print ("math.log(100.12) : ", math.log(100.12)) print ("math.log(100.72) : ", math.log(100.72)) print ("math.log(math.pi) : ", math.log(math.pi))
When we run the above program, it produces the following output −
math.log(100.12) : 4.6063694665635735 math.log(100.72) : 4.612344389736092 math.log(math.pi) : 1.1447298858494002
log10() Function
The log10() function returns base-10 logarithm of x for x > 0.
Syntax
Following is the syntax for log10() function −
import math math.log10(x)
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This function returns the base-10 logarithm of x for x > 0.
Example
The following example shows the usage of the log10() function.
import math # This will import math module print ("math.log10(100.12) : ", math.log10(100.12)) print ("math.log10(100.72) : ", math.log10(100.72)) print ("math.log10(119) : ", math.log10(119)) print ("math.log10(math.pi) : ", math.log10(math.pi))
When we run the above program, it produces the following output −
math.log10(100.12) : 2.0005208409361854 math.log10(100.72) : 2.003115717099806 math.log10(119) : 2.0755469613925306 math.log10(math.pi) : 0.49714987269413385
log1p() Function
The log1p() function in math module returns the natural logarithm of 1+x (base e). The result is calculated in a way which is accurate for x near zero.
Syntax
math.log1p(x)
Parameters
x − int or float operand.
Return value
The function returns natural log of 1+x.
Example
from math import log1p x = 4 val = log1p(x) print ("x: ",x, "log1p(x): ", val) x = 2.5 val = log1p(x) print ("x: ",x, "log1p(x): ", val) x = -3 val = log1p(x) print ("x: ",x, "log1p(x): ", val)
It will produce the following output −
x: 4 log1p(x): 1.6094379124341003 x: 2.5 log1p(x): 1.252762968495368 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 12, in <module> val = log1p(x) ^^^^^^^^ ValueError: math domain error
Negative value of x raises ValueError
log2() Function
The log2() function in math module returns the base-2 logarithm of x. This is usually more accurate than log(x, 2).
Syntax
math.log2(x)
Parameters
x − int or float operand
Return Value
The function returns base-2 log of x.
Example
from math import log2 x = 4 val = log2(x) print ("x: ",x, "log2(x): ", val) x = 2.5 val = log2(x) print ("x: ",x, "log2(x): ", val) x = -3 val = log2(x) print ("x: ",x, "log2(x): ", val)
It will produce the following output −
x: 4 log2(x): 2.0 x: 2.5 log2(x): 1.3219280948873624 Traceback (most recent call last): File "C:\Users\mlath\examples\main.py", line 12, in <module> val = log2(x) ^^^^^^^ ValueError: math domain error
pow() Function
The pow() function value of x raised to y. math.pow() converts both its arguments to type float. Use ** or the built-in pow() function for computing exact integer powers.
Syntax
Following is the syntax for pow() function −
import math math.pow( x,y )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x, y − This is a numeric expression.
Return Value
This function returns value of x raised to y.
Example
The following example shows the usage of the pow() function −
import math # This will import math module print ("math.pow(100, 2) : ", math.pow(100, 2)) print ("math.pow(100, -2) : ", math.pow(100, -2)) print ("math.pow(2, 4) : ", math.pow(2, 4)) print ("math.pow(3, 0) : ", math.pow(3, 0))
It will produce the following output −
math.pow(100, 2) : 10000.0 math.pow(100, -2) : 0.0001 math.pow(2, 4) : 16.0 math.pow(3, 0) : 1.0
sqrt() Function
The sqrt() function returns the square root of x for x > 0.
Syntax
Following is the syntax for sqrt() function −
import math math.sqrt( x )
Note − This function is not accessible directly, so we need to import the math module and then we need to call this function using the math static object.
Parameters
x − This is a numeric expression.
Return Value
This method returns square root of x for x > 0.
Example
The following example shows the usage of sqrt() function −
import math # This will import math module print ("math.sqrt(100) : ", math.sqrt(100)) print ("math.sqrt(7) : ", math.sqrt(7)) print ("math.sqrt(math.pi) : ", math.sqrt(math.pi))
When we run the above program, it produces the following output −
math.sqrt(100) : 10.0 math.sqrt(7) : 2.6457513110645907 math.sqrt(math.pi) : 1.7724538509055159