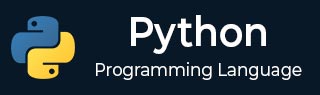
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python os.path.sameopenfile() Method
The Python os.path.sameopenfile() method is used to check whether two file descriptors refer to the same file or directory in the file system.
If both file descriptors refer to the same open file or directory (even if they are different descriptors), the method returns True. If the file descriptors do not refer to the same open file or directory, it returns False.
Syntax
Following is the basic syntax of the Python os.path.sameopenfile() method −
os.path.sameopenfile(fp1, fp2)
Parameter
This method accepts the following parameters −
fp1 − This is the file descriptor of the first file to be compared.
fp2 − This is the file descriptor of the second file to be compared.
Return Value
The method returns a boolean value "True" or "False". It returns True if both file descriptors refer to the same file, otherwise it returns False.
Example
In the following example, we are opening the same file "C://Users//Lenovo//Desktop//file.txt" twice with different file descriptors "fd1" and "fd2". We are then checking if both file descriptors refer to the same file using the sameopenfile() method −
import os fd1 = os.open("C://Users//Lenovo//Desktop//file.txt", os.O_RDONLY) fd2 = os.open("C://Users//Lenovo//Desktop//file.txt", os.O_RDONLY) result = os.path.sameopenfile(fd1, fd2) print("The result obtained is:",result)
Output
Following is the output of the above code −
The result obtained is: True
Example
Here, we open two different files with different file descriptors "fd1" and "fd2". We then check if both file descriptors refer to the same file or not −
import os fd1 = os.open("C://Users//Lenovo//Desktop//file.txt", os.O_RDONLY) fd2 = os.open("C://Users//Lenovo//Desktop//file2.txt", os.O_RDONLY) result = os.path.sameopenfile(fd1, fd2) print("The result obtained is:",result)
Output
Output of the above code is as follows −
The result obtained is: False
Example
This example opens the file "file.txt" with a file descriptor "fd1", closes "fd1", opens the same file again with a new file descriptor "fd2", and checks if both file descriptors refer to the same file.
Even though "fd1" is closed, its file descriptor is still associated with the same file until the operating system reuses it, so the output is True" −
import os fd1 = os.open("C://Users//Lenovo//Desktop//file.txt", os.O_RDONLY) os.close(fd1) fd2 = os.open("C://Users//Lenovo//Desktop//file.txt", os.O_RDONLY) result = os.path.sameopenfile(fd1, fd2) print("The result obtained is:",result)
Output
The result produced is as follows −
The result obtained is: True
Example
Now, we open two files with file descriptors "fd1" and "fd2", then check whether both file descriptors refer to the same file. Since both file do not exist, the method returns "FileNotFoundError" −
import os fd1 = os.open("/home/lenovo/documents/file.txt", os.O_RDONLY) fd2 = os.open("/home/lenovo/documents/file.txt", os.O_RDONLY) result = os.path.sameopenfile(fd1, fd2) print("The result obtained is:",result)
Output
We get the output as shown below −
Traceback (most recent call last): File "C:\Users\Lenovo\Desktop\untitled.py", line 2, in <module> fd1 = os.open("/home/lenovo/documents/file.txt", os.O_RDONLY) FileNotFoundError: [Errno 2] No such file or directory: '/home/lenovo/documents/file.txt'