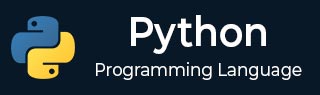
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - String Methods
Python's built-in str class defines different methods. They help in manipulating strings. Since string is an immutable object, these methods return a copy of the original string, performing the respective processing on it. The string methods can be classified in following categories −
Case Conversion Methods
This category of built-in methods of Python's str class deal with the conversion of alphabet characters in the string object. Following methods fall in this category −
Sr.No. | Method & Description |
---|---|
1 |
Capitalizes first letter of string |
2 |
Converts all uppercase letters in string to lowercase. Similar to lower(), but works on UNICODE characters alos |
3 |
Converts all uppercase letters in string to lowercase. |
4 |
Inverts case for all letters in string. |
5 |
Returns "titlecased" version of string, that is, all words begin with uppercase and the rest are lowercase. |
6 |
Converts lowercase letters in string to uppercase. |
Alignment Methods
Following methods in the str class control the alignment of characters within the string object.
Sr.No. | Methods & Description |
---|---|
1 |
Returns a string padded with fillchar with the original string centered to a total of width columns. |
2 |
Returns a space-padded string with the original string left-justified to a total of width columns. |
3 |
Returns a space-padded string with the original string right-justified to a total of width columns. |
4 |
Expands tabs in string to multiple spaces; defaults to 8 spaces per tab if tabsize not provided. |
5 |
Returns original string leftpadded with zeros to a total of width characters; intended for numbers, zfill() retains any sign given (less one zero). |
Split and Join Methods
Python has the following methods to perform split and join operations −
Sr.No. | Method & Description |
---|---|
1 |
Removes all leading whitespace in string. |
2 |
Removes all trailing whitespace of string. |
3 |
Performs both lstrip() and rstrip() on string |
4 |
Splits the string from the end and returns a list of substrings |
5 |
Splits string according to delimiter (space if not provided) and returns list of substrings. |
6 |
Splits string at NEWLINEs and returns a list of each line with NEWLINEs removed. |
7 |
Splits the string in three string tuple at the first occurrence of separator |
8 |
Splits the string in three string tuple at the ladt occurrence of separator |
9 |
Concatenates the string representations of elements in sequence into a string, with separator string. |
10 |
Returns a string after removing the prefix string |
11 |
Returns a string after removing the suffix string |
Boolean String Methods
Following methods in str class return True or False.
Sr.No. | Methods & Description |
---|---|
1 |
Returns true if string has at least 1 character and all characters are alphanumeric and false otherwise. |
2 |
Returns true if string has at least 1 character and all characters are alphabetic and false otherwise. |
3 |
Returns true if the string contains only digits and false otherwise. |
4 |
Returns true if string has at least 1 cased character and all cased characters are in lowercase and false otherwise. |
5 |
Returns true if a unicode string contains only numeric characters and false otherwise. |
6 |
Returns true if string contains only whitespace characters and false otherwise. |
7 |
Returns true if string is properly "titlecased" and false otherwise. |
8 |
Returns true if string has at least one cased character and all cased characters are in uppercase and false otherwise. |
9 |
Returns True is all the characters in the string are from the ASCII character set |
10 |
Checks if all the characters are decimal characters |
11 |
Checks whether the string is a valid Python identifier |
12 |
Checks whether all the characters in the string are printable |
Find and Replace Methods
Following are the Find and Replace methods in Python −
Sr.No. | Method & Description |
---|---|
1 |
Counts how many times sub occurs in string or in a substring of string if starting index beg and ending index end are given. |
2 |
Determine if sub occurs in string or in a substring of string if starting index beg and ending index end are given returns index if found and -1 otherwise. |
3 |
Same as find(), but raises an exception if str not found. |
4 |
Replaces all occurrences of old in string with new or at most max occurrences if max given. |
5 |
Same as find(), but search backwards in string. |
6 |
Same as index(), but search backwards in string. |
7 |
Determines if string or a substring of string (if starting index beg and ending index end are given) starts with substring sub; returns true if so and false otherwise. |
8 |
Determines if string or a substring of string (if starting index beg and ending index end are given) ends with suffix; returns true if so and false otherwise. |
Translation Methods
Following are the Translation methods of the string −
Sr.No. | Method & Description |
---|---|
1 |
Returns a translation table to be used in translate function. |
2 |
translate(table, deletechars="") Translates string according to translation table str(256 chars), removing those in the del string. |