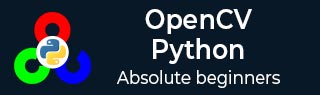
- OpenCV Python Tutorial
- OpenCV Python - Home
- OpenCV Python - Overview
- OpenCV Python - Environment
- OpenCV Python - Reading Image
- OpenCV Python - Write Image
- OpenCV Python - Using Matplotlib
- OpenCV Python - Image Properties
- OpenCV Python - Bitwise Operations
- OpenCV Python - Shapes and Text
- OpenCV Python - Mouse Events
- OpenCV Python - Add Trackbar
- OpenCV Python - Resize and Rotate
- OpenCV Python - Image Threshold
- OpenCV Python - Image Filtering
- OpenCV Python - Edge Detection
- OpenCV Python - Histogram
- OpenCV Python - Color Spaces
- OpenCV Python - Transformations
- OpenCV Python - Image Contours
- OpenCV Python - Template Matching
- OpenCV Python - Image Pyramids
- OpenCV Python - Image Addition
- OpenCV Python - Image Blending
- OpenCV Python - Fourier Transform
- OpenCV Python - Capture Videos
- OpenCV Python - Play Videos
- OpenCV Python - Images From Video
- OpenCV Python - Video from Images
- OpenCV Python - Face Detection
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - Feature Detection
- OpenCV Python - Feature Matching
- OpenCV Python - Digit Recognition
- OpenCV Python Resources
- OpenCV Python - Quick Guide
- OpenCV Python - Resources
- OpenCV Python - Discussion
OpenCV Python - Template Matching
The technique of template matching is used to detect one or more areas in an image that matches with a sample or template image.
Cv.matchTemplate() function in OpenCV is defined for the purpose and the command for the same is as follows:
cv.matchTemplate(image, templ, method)
Where image is the input image in which the templ (template) pattern is to be located. The method parameter takes one of the following values −
- cv.TM_CCOEFF,
- cv.TM_CCOEFF_NORMED, cv.TM_CCORR,
- cv.TM_CCORR_NORMED,
- cv.TM_SQDIFF,
- cv.TM_SQDIFF_NORMED
This method slides the template image over the input image. This is a similar process to convolution and compares the template and patch of input image under the template image.
It returns a grayscale image, whose each pixel denotes how much it matches with the template. If the input image is of size (WxH) and template image is of size (wxh), the output image will have a size of (W-w+1, H-h+1). Hence, that rectangle is your region of template.
Example
In an example below, an image having Indian cricketer Virat Kohli’s face is used as a template to be matched with another image which depicts his photograph with another Indian cricketer M.S.Dhoni.
Following program uses a threshold value of 80% and draws a rectangle around the matching face −
import cv2 import numpy as np img = cv2.imread('Dhoni-and-Virat.jpg',1) cv2.imshow('Original',img) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) template = cv2.imread('virat.jpg',0) cv2.imshow('Template',template) w,h = template.shape[0], template.shape[1] matched = cv2.matchTemplate(gray,template,cv2.TM_CCOEFF_NORMED) threshold = 0.8 loc = np.where( matched >= threshold) for pt in zip(*loc[::-1]): cv2.rectangle(img, pt, (pt[0] + w, pt[1] + h), (0,255,255), 2) cv2.imshow('Matched with Template',img)
Output
The original image, the template and matched image of the result as follows −
Original image
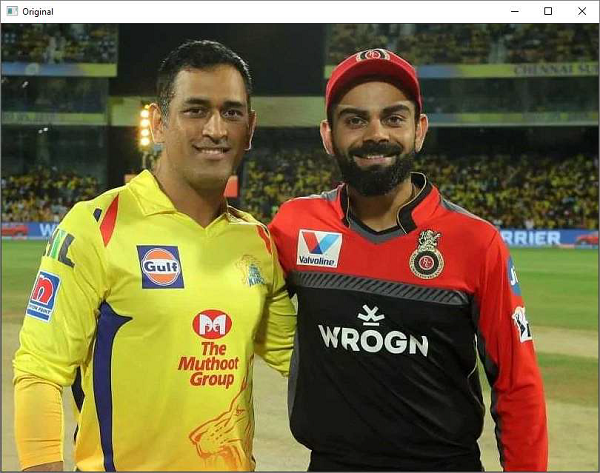
The template is as follows −
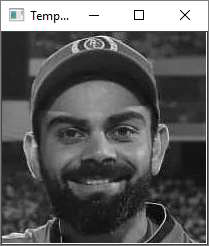
The image when matched with template is as follows −
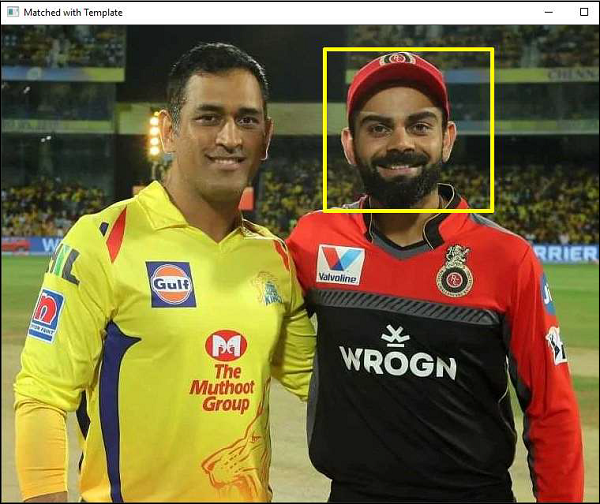
To Continue Learning Please Login