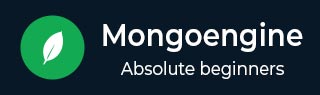
- MongoEngine Tutorial
- MongoEngine - Home
- MongoEngine - MongoDB
- MongoEngine - MongoDB Compass
- MongoEngine - Object Document Mapper
- MongoEngine - Installation
- MongoEngine - Connecting to MongoDB Database
- MongoEngine - Document Class
- MongoEngine - Dynamic Schema
- MongoEngine - Fields
- MongoEngine - Add/Delete Document
- MongoEngine - Querying Database
- MongoEngine - Filters
- MongoEngine - Query Operators
- MongoEngine - QuerySet Methods
- MongoEngine - Sorting
- MongoEngine - Custom Query Sets
- MongoEngine - Indexes
- MongoEngine - Aggregation
- MongoEngine - Advanced Queries
- MongoEngine - Document Inheritance
- MongoEngine - Atomic Updates
- MongoEngine - Javascript
- MongoEngine - GridFS
- MongoEngine - Signals
- MongoEngine - Text Search
- MongoEngine - Extensions
- MongoEngine Useful Resources
- MongoEngine - Quick Guide
- MongoEngine - Useful Resources
- MongoEngine - Discussion
MongoEngine - Querying Database
The connect() function returns a MongoClient object. Using list_database_names() method available to this object, we can retrieve number of databases on the server.
from mongoengine import * con=connect('newdb') dbs=con.list_database_names() for db in dbs: print (db)
It is also possible to obtain list of collections in a database, using list_collection_names() method.
collections=con['newdb'].list_collection_names() for collection in collections: print (collection)
As mentioned earlier, the Document class has objects attribute that enable access to objects associated with the database.
The newdb database has a products collection corresponding to Document class below. To get all documents, we use objects attribute as follows −
from mongoengine import * con=connect('newdb') class products (Document): ProductID=IntField(required=True) Name=StringField() price=IntField() for product in products.objects: print ('ID:',product.ProductID, 'Name:',product.Name, 'Price:',product.price)
Output
ID: 1 Name: Laptop Price: 25000 ID: 2 Name: TV Price: 50000 ID: 3 Name: Router Price: 2000 ID: 4 Name: Scanner Price: 5000 ID: 5 Name: Printer Price: 12500
Advertisements