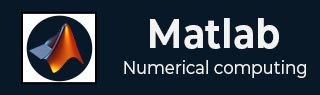
- Matlab Tutorial
- MATLAB - Home
- MATLAB - Overview
- MATLAB - Environment Setup
- MATLAB - Syntax
- MATLAB - Variables
- MATLAB - Commands
- MATLAB - M-Files
- MATLAB - Data Types
- MATLAB - Operators
- MATLAB - Decisions
- MATLAB - Loops
- MATLAB - Vectors
- MATLAB - Matrix
- MATLAB - Arrays
- MATLAB - Colon Notation
- MATLAB - Numbers
- MATLAB - Strings
- MATLAB - Functions
- MATLAB - Data Import
- MATLAB - Data Output
- MATLAB Advanced
- MATLAB - Plotting
- MATLAB - Graphics
- MATLAB - Algebra
- MATLAB - Calculus
- MATLAB - Differential
- MATLAB - Integration
- MATLAB - Polynomials
- MATLAB - Transforms
- MATLAB - GNU Octave
- MATLAB - Simulink
- MATLAB Useful Resources
- MATLAB - Quick Guide
- MATLAB - Useful Resources
- MATLAB - Discussion
MATLAB - Arithmetic Operations
MATLAB allows two different types of arithmetic operations −
- Matrix arithmetic operations
- Array arithmetic operations
Matrix arithmetic operations are same as defined in linear algebra. Array operations are executed element by element, both on one dimensional and multi-dimensional array.
The matrix operators and arrays operators are differentiated by the period (.) symbol. However, as the addition and subtraction operation is same for matrices and arrays, the operator is same for both cases.
The following table gives brief description of the operators −
Sr.No. | Operator & Description |
---|---|
1 | + Addition or unary plus. A+B adds the values stored in variables A and B. A and B must have the same size, unless one is a scalar. A scalar can be added to a matrix of any size. |
2 | - Subtraction or unary minus. A-B subtracts the value of B from A. A and B must have the same size, unless one is a scalar. A scalar can be subtracted from a matrix of any size. |
3 | * Matrix multiplication. C = A*B is the linear algebraic product of the matrices A and B. More precisely, For non-scalar A and B, the number of columns of A must be equal to the number of rows of B. A scalar can multiply a matrix of any size. |
4 | .* Array multiplication. A.*B is the element-by-element product of the arrays A and B. A and B must have the same size, unless one of them is a scalar. |
5 | / Slash or matrix right division. B/A is roughly the same as B*inv(A). More precisely, B/A = (A'\B')'. |
6 | ./ Array right division. A./B is the matrix with elements A(i,j)/B(i,j). A and B must have the same size, unless one of them is a scalar. |
7 | \ Backslash or matrix left division. If A is a square matrix, A\B is roughly the same as inv(A)*B, except it is computed in a different way. If A is an n-by-n matrix and B is a column vector with n components, or a matrix with several such columns, then X = A\B is the solution to the equation AX = B. A warning message is displayed if A is badly scaled or nearly singular. |
8 | .\ Array left division. A.\B is the matrix with elements B(i,j)/A(i,j). A and B must have the same size, unless one of them is a scalar. |
9 | ^ Matrix power. X^p is X to the power p, if p is a scalar. If p is an integer, the power is computed by repeated squaring. If the integer is negative, X is inverted first. For other values of p, the calculation involves eigenvalues and eigenvectors, such that if [V,D] = eig(X), then X^p = V*D.^p/V. |
10 | .^ Array power. A.^B is the matrix with elements A(i,j) to the B(i,j) power. A and B must have the same size, unless one of them is a scalar. |
11 | ' Matrix transpose. A' is the linear algebraic transpose of A. For complex matrices, this is the complex conjugate transpose. |
12 | .' Array transpose. A.' is the array transpose of A. For complex matrices, this does not involve conjugation. |
Example
The following examples show the use of arithmetic operators on scalar data. Create a script file with the following code −
a = 10; b = 20; c = a + b d = a - b e = a * b f = a / b g = a \ b x = 7; y = 3; z = x ^ y
When you run the file, it produces the following result −
c = 30 d = -10 e = 200 f = 0.50000 g = 2 z = 343
Functions for Arithmetic Operations
Apart from the above-mentioned arithmetic operators, MATLAB provides the following commands/functions used for similar purpose −
Sr.No. | Function & Description |
---|---|
1 | uplus(a) Unary plus; increments by the amount a |
2 | plus (a,b) Plus; returns a + b |
3 | uminus(a) Unary minus; decrements by the amount a |
4 | minus(a, b) Minus; returns a - b |
5 | times(a, b) Array multiply; returns a.*b |
6 | mtimes(a, b) Matrix multiplication; returns a* b |
7 | rdivide(a, b) Right array division; returns a ./ b |
8 | ldivide(a, b) Left array division; returns a.\ b |
9 | mrdivide(A, B) Solve systems of linear equations xA = B for x |
10 | mldivide(A, B) Solve systems of linear equations Ax = B for x |
11 | power(a, b) Array power; returns a.^b |
12 | mpower(a, b) Matrix power; returns a ^ b |
13 | cumprod(A) Cumulative product; returns an array of the same size as the array A containing the cumulative product.
|
14 | cumprod(A, dim) Returns the cumulative product along dimension dim. |
15 | cumsum(A) Cumulative sum; returns an array A containing the cumulative sum.
|
16 | cumsum(A, dim) Returns the cumulative sum of the elements along dimension dim. |
17 | diff(X) Differences and approximate derivatives; calculates differences between adjacent elements of X.
|
18 | diff(X,n) Applies diff recursively n times, resulting in the nth difference. |
19 | diff(X,n,dim) It is the nth difference function calculated along the dimension specified by scalar dim. If order n equals or exceeds the length of dimension dim, diff returns an empty array. |
20 | prod(A) Product of array elements; returns the product of the array elements of A.
The prod function computes and returns B as single if the input, A, is single. For all other numeric and logical data types, prod computes and returns B as double. |
21 | prod(A,dim) Returns the products along dimension dim. For example, if A is a matrix, prod(A,2) is a column vector containing the products of each row. |
22 | prod(___,datatype) multiplies in and returns an array in the class specified by datatype. |
23 | sum(A)
|
24 | sum(A,dim) Sums along the dimension of A specified by scalar dim. |
25 | sum(..., 'double') sum(..., dim,'double') Perform additions in double-precision and return an answer of type double, even if A has data type single or an integer data type. This is the default for integer data types. |
26 | sum(..., 'native') sum(..., dim,'native') Perform additions in the native data type of A and return an answer of the same data type. This is the default for single and double. |
27 | ceil(A) Round toward positive infinity; rounds the elements of A to the nearest integers greater than or equal to A. |
28 | fix(A) Round toward zero |
29 | floor(A) Round toward negative infinity; rounds the elements of A to the nearest integers less than or equal to A. |
30 | idivide(a, b) idivide(a, b,'fix') Integer division with rounding option; is the same as a./b except that fractional quotients are rounded toward zero to the nearest integers. |
31 | idivide(a, b, 'round') Fractional quotients are rounded to the nearest integers. |
32 | idivide(A, B, 'floor') Fractional quotients are rounded toward negative infinity to the nearest integers. |
33 | idivide(A, B, 'ceil') Fractional quotients are rounded toward infinity to the nearest integers. |
34 | mod (X,Y) Modulus after division; returns X - n.*Y where n = floor(X./Y). If Y is not an integer and the quotient X./Y is within round off error of an integer, then n is that integer. The inputs X and Y must be real arrays of the same size, or real scalars (provided Y ~=0). Please note −
|
35 | rem (X,Y) Remainder after division; returns X - n.*Y where n = fix(X./Y). If Y is not an integer and the quotient X./Y is within roundoff error of an integer, then n is that integer. The inputs X and Y must be real arrays of the same size, or real scalars(provided Y ~=0). Please note that −
|
36 | round(X) Round to nearest integer; rounds the elements of X to the nearest integers. Positive elements with a fractional part of 0.5 round up to the nearest positive integer. Negative elements with a fractional part of -0.5 round down to the nearest negative integer. |