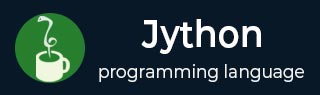
- Jython Tutorial
- Jython - Home
- Jython - Overview
- Jython - Installation
- Jython - Importing Java Libraries
- Jython - Variables and Data Types
- Jython - Using Java Collection Types
- Jython - Decision Control
- Jython - Loops
- Jython - Functions
- Jython - Modules
- Jython - Package
- Jython - Java Application
- Jython - Eclipse Plugin
- Jython - A Project in Eclipse
- Jython - NetBeans Plugin & Project
- Jython - Servlets
- Jython - JDBC
- Jython - Using the Swing GUI library
- Jython - Layout Management
- Jython - Event Handling
- Jython - Menus
- Jython - Dialogs
- Jython Useful Resources
- Jython - Quick Guide
- Jython - Useful Resources
- Jython - Discussion
Jython - Functions
A complex programming logic is broken into one or more independent and reusable blocks of statements called as functions. Python’s standard library contains large numbers of built-in functions. One can also define their own function using the def keyword. User defined name of the function is followed by a block of statements that forms its body, which ends with the return statement.
Once defined, it can be called from any environment any number of times. Let us consider the following code to make the point clear.
#definition of function defSayHello(): "optional documentation string" print "Hello World" return #calling the function SayHello()
A function can be designed to receive one or more parameters / arguments from the calling environment. While calling such a parameterized function, you need to provide the same number of parameters with similar data types used in the function definition, otherwise Jython interpreter throws a TypeError exception.
Example
#defining function with two arguments def area(l,b): area = l*b print "area = ",area return #calling function length = 10 breadth = 20 #with two arguments. This is OK area(length, breadth) #only one argument provided. This will throw TypeError area(length)
The output will be as follows −
area = 200 Traceback (most recent call last): File "area.py", line 11, in <module> area(length) TypeError: area() takes exactly 2 arguments (1 given)
After performing the steps defined in it, the called function returns to the calling environment. It can return the data, if an expression is mentioned in front of the return keyword inside the definition of the function.
#defining function def area(l,b): area = l*b print "area = ",area return area #calling function length = 10 breadth = 20 #calling function and obtaining its reurned value result = area(length, breadth) print "value returned by function : ", result
The following output is obtained if the above script is executed from the Jython prompt.
area = 200 value returned by function : 200