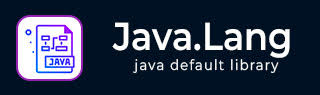
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Thread.getUncaughtExceptionHandler() Method
Description
The Java Thread getUncaughtExceptionHandler() method returns the handler invoked when this thread abruptly terminates due to an uncaught exception.
Declaration
Following is the declaration for java.lang.Thread.getUncaughtExceptionHandler() method
public Thread.UncaughtExceptionHandler getUncaughtExceptionHandler()
Parameters
NA
Return Value
This method does not return any value.
Exception
NA
Example: Getting UncaughtExceptionHandler for a Thread
The following example shows the usage of Java Thread getUncaughtExceptionHandler() method. In this program, we've created a thread class AdminThread by implementing Runnable interface. In run method, we're throwing a RuntimeException. In main method, we've created a new thread of AdminThread class and using setUncaughtExceptionHandler() method, we've set a uncaught exception handler which prints the exception raised. Now using getUncaughtExceptionHandler(), the exception handler is retrieved and printed.
Using start() method, we've started the thread and result is printed on the console.
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = new Thread(new AdminThread()); t.setUncaughtExceptionHandler(new Thread.UncaughtExceptionHandler() { public void uncaughtException(Thread t, Throwable e) { System.out.println(t + " throws exception: " + e); } }); // this will call run() function t.start(); /* returns the handler invoked when this thread abruptly terminates due to an uncaught exception. */ Thread.UncaughtExceptionHandler handler = t.getUncaughtExceptionHandler(); System.out.println(handler); } } class AdminThread implements Runnable { public void run() { throw new RuntimeException(); } }
Output
Let us compile and run the above program, this will produce the following result −
com.tutorialspoint.ThreadDemo$1@28a418fc Thread[#21,Thread-0,5,main] throws exception: java.lang.RuntimeException