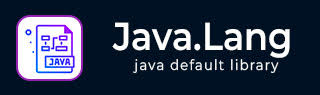
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java.lang.Thread.getStackTrace() Method
Description
The java.lang.Thread.getStackTrace() method returns an array of stack trace elements representing the stack dump of this thread.This will return a zero-length array if this thread has not started or has terminated.
If the returned array is of non-zero length then the first element of the array represents the top of the stack, which is the most recent method invocation in the sequence. The last element of the array represents the bottom of the stack, which is the least recent method invocation in the sequence.
Declaration
Following is the declaration for java.lang.Thread.getStackTrace() method
public StackTraceElement[] getStackTrace()
Parameters
NA
Return Value
This method returns an array of StackTraceElement, each represents one stack frame.
Exception
SecurityException − if a security manager exists and its checkPermission method doesn't allow getting the stack trace of thread.
Example
The following example shows the usage of java.lang.Thread.getStackTrace() method.
package com.tutorialspoint; import java.lang.*; public class ThreadDemo { public static void main(String[] args) { function(); } public static void function() { new ThreadDemo().function2(); } public void function2() { System.out.println(Thread.currentThread().getStackTrace()[3].getClassName()); } }
Let us compile and run the above program, this will produce the following result −
com.tutorialspoint.ThreadDemo
To Continue Learning Please Login