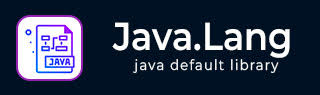
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Thread checkAccess() Method
Description
The Java Thread checkAccess() method determines if the currently running thread has permission to modify this thread.
Declaration
Following is the declaration for java.lang.Thread.checkAccess() method
public final void checkAccess()
Parameters
NA
Return Value
This method does not return any value.
Exception
SecurityException − if the current thread is not allowed to access this thread.
Example: Checking if a Thread has Access in Multi-Threaded Environment
The following example shows the usage of Java Thread checkAccess() method. In this program, we've created a thread class ThreadClass by implementing Runnable interface. In main method, we've created the instance of ThreadClass. Then using currentThread() method, current thread is retrieved and using checkAccess() method, we've checked if current thread has permission or not.
package com.tutorialspoint; public class ThreadDemo { public static void main(String args[]) { new ThreadClass("A"); Thread t = Thread.currentThread(); try { /* determines if the currently running thread has permission to modify this thread */ t.checkAccess(); System.out.println("You have permission to modify"); } /* if the current thread is not allowed to access this thread, then it result in throwing a SecurityException. */ catch(Exception e) { System.out.println(e); } } } class ThreadClass implements Runnable { Thread t; String str; ThreadClass(String str) { this.str = str; t = new Thread(this); // this will call run() function t.start(); } public void run() { System.out.println("This is run() function"); } }
Output
Let us compile and run the above program, this will produce the following result −
You have permission to modify This is run() function
Example: Checking if a Thread has Access in Single-Threaded Environment
The following example shows the usage of Java Thread checkAccess() method. In this program, we've created a class ThreadDemo. In main method, using currentThread() method, current thread is retrieved and using checkAccess() method, we've checked if current thread has permission or not.
package com.tutorialspoint; public class ThreadDemo { public static void main(String args[]) { Thread t = Thread.currentThread(); try { /* determines if the currently running thread has permission to modify this thread */ t.checkAccess(); System.out.println("You have permission to modify"); } /* if the current thread is not allowed to access this thread, then it result in throwing a SecurityException. */ catch(Exception e) { System.out.println(e); } } }
Output
Let us compile and run the above program, this will produce the following result −
You have permission to modify