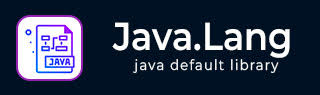
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java System setProperties() Method
Description
The Java System setProperties() method sets the system properties to the Properties argument.
Declaration
Following is the declaration for java.lang.System.setProperties() method
public static void setProperties(Properties props)
Parameters
props − This is the new system properties.
Return Value
This method does not return any value.
Exception
SecurityException − if a security manager exists and its checkPropertiesAccess method doesn't allow access to the system properties.
Example: Clearing User Directory and Setting New Path
The following example shows the usage of Java System setProperties() method. In this example, we've cleared the current user.dir system property using System.clearProperty() method. We've used the returned value of clearProperty() to print the previous user.dir property value. Using setProperties() method, a new path is set as user.dir via properties object and using getProperty(), this updated property is retrieved and printed.
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { // returns the previous string value of the system property String userDir = System.clearProperty("user.dir"); System.out.println("Previous user.dir = " + userDir); // sets the new property Properties p = System.getProperties(); p.put("user.dir", "C:/tutorialspoint/java"); System.setProperties(p); // gets the system property after changes done by setProperty System.out.println("Current user.dir = " + System.getProperty("user.dir")); } }
Output
Let us compile and run the above program, this will produce the following result −
Previous user.dir = C:\Users\Tutorialspoint\eclipse-workspace\Tutorialspoint Current user.dir = C:/tutorialspoint/java
Example: Clearing Java Class Path and Setting New Path
The following example shows the usage of Java System setProperties() method. In this example, we've cleared the current java.class.path system property using System.clearProperty() method. We've used the returned value of clearProperty() to print the previous java.class.path property value. Using setProperties() method, a new path is set as java.class.path via properties object and using getProperty(), this updated property is retrieved and printed.
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { // returns the previous string value of the system property String classPath = System.clearProperty("java.class.path"); System.out.println("Previous Class Path = " + classPath); // sets the new property Properties p = System.getProperties(); p.put("java.class.path", "C:/tutorialspoint/java"); System.setProperties(p); // gets the system property after changes done by setProperty System.out.println("Current Class Path = " + System.getProperty("java.class.path")); } }
Output
Let us compile and run the above program, this will produce the following result −
Previous Class Path = C:\Users\Tutorialspoint\eclipse-workspace\Tutorialspoint\bin;C:\Users\Tutorialspoint\eclipse-workspace\Tutorialspoint\lib\jmh-core-1.37.jar;C:\Users\Tutorialspoint\eclipse-workspace\Tutorialspoint\lib\jmh-generator-annprocess-1.37.jar;C:\Users\Tutorialspoint\eclipse-workspace\Tutorialspoint\lib Current Class Path = C:/tutorialspoint/java
Example: Clearing Non-Existing Property and Setting New Value
The following example shows the usage of Java System setProperties() method. In this example, we've cleared the current tmp.dir system property using System.clearProperty() method. We've used the returned value of clearProperty() to print the previous tmp.dir property value as null. Using setProperties() method, a new path is set as tmp.dir via properties object and using getProperty(), this updated property is retrieved and printed.
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { // returns the previous string value of the system property String tmpDir = System.clearProperty("tmp.dir"); System.out.println("Previous Temporary Dir = " + tmpDir); // sets the new property Properties p = System.getProperties(); p.put("tmp.dir", "C:/tutorialspoint/java"); System.setProperties(p); // gets the system property after changes done by setProperty System.out.println("Current Temporary Dir = " + System.getProperty("tmp.dir")); } }
Output
Let us compile and run the above program, this will produce the following result −
Previous Temporary Dir = null Current Temporary Dir = C:/tutorialspoint/java