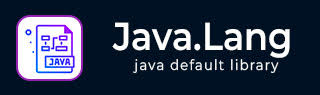
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java System exit() Method
Description
The java System exit() method terminates the currently running Java Virtual Machine.
The argument serves as a status code; by convention, a nonzero status code indicates abnormal termination.
Declaration
Following is the declaration for java.lang.System.exit() method
public static void exit(int status)
Parameters
status − This is the exit status.
Return Value
This method does not return any value.
Exception
SecurityException − if a security manager exists and its checkExit method doesn't allow exit with the specified status.
Example: Terminating a program on Conditional Basis
The following example shows the usage of Java System exit() method. In this program, we've created two arrays of ints and initialized them with some values. Now using System.arraycopy() method, the first element of the first array arr1 is copied to second array at index 0. Then we've printed the second array to show the updated array as result. In next for loop statement, we've used the exit() statement while checking the value of array element being greater than 20. So only three elements of array2 are printed and program exits.
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { int arr1[] = { 0, 1, 2, 3, 4, 5 }; int arr2[] = { 0, 10, 20, 30, 40, 50 }; int i; // copies an array from the specified source array System.arraycopy(arr1, 0, arr2, 0, 1); System.out.print("array2 = "); for(int i= 0; i < arr2.length; i++) { System.out.print(arr2[i] + " "); } for(i = 0;i < 3;i++) { if(arr2[i] > = 20) { System.out.println("exit..."); System.exit(0); } else { System.out.println("arr2["+i+"] = " + arr2[i]); } } } }
Output
Let us compile and run the above program, this will produce the following result −
array2 = 0 10 20 30 40 50 60 arr2[0] = 0 arr2[1] = 10 exit...