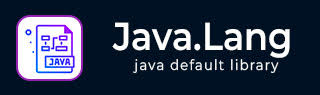
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - String toString() Method
This method is overridden from the class Object. Usually the toString() method returns the string representation of the current object. The Java String toString() method is used to retrieve a string by itself and represents the result in text format. Therefore, no actual conversion is carried out here.
A string in Java is a group of characters that is an object of the Java lang class. The string class in Java is used to create and modify strings. A string cannot have its value modified after it has been created. There is no need to call this method on a string object explicitly, it just returns the current string without making any changes. Typically this method invoked implicitly.
Syntax
Following is the syntax for Java String toString() method −
public String toString()
Parameters
This method does not accept any parameter.
Return Value
This method returns the string itself.
Example
In the example given below a String object is created with the value "Welcome to Tutorials Point". Then, we are invoking the toString() method on the created string −
import java.io.*; public class StringDemo { public static void main(String args[]) { String obj = new String("Welcome to Tutorials Point"); System.out.println("The string is: " + obj.toString()); } }
Output
If you compile and run the program above, the output will be displayed as follows −
The string is: Welcome to Tutorials Point
Example
The following example shows the usage of Java String toString() method to convert an integer to its equivalent string value −
import java.lang.*; public class StringDemo { public static void main(String[] args) { // converts integer value in String representation int val1 = 325; String str1 = Integer.toString(val1); System.out.println("String representation of integer value = " + str1); } }
Output
If you compile and run the above program, it will produce the following result −
String representation of integer value = 325
Example
Below is an example to convert a double value to its equivalent string using toString() method in Java −
import java.lang.*; public class StringDemo { public static void main(String[] args) { // converts double value in String representation double val2 = 876.23; String str2 = Double.toString(val2); System.out.println("String representation of double value = " + str2); } }
Output
On executing the program above, the output is obtained as follows −
String representation of double value = 876.23
Example
In the code below, we are defining a class with name Vehicle and creating two objects 'v1' and 'v2' of it. Since the toString() method in Java works on objects when the value of 'v1' and 'v2' is printed, this method is called implicitly.
class Vehicle { String brand; Integer modelNumber; Vehicle(String brand, Integer modelNumber) { // constructor to initialize variables of the class this.brand = brand; this.modelNumber = modelNumber; } } public class StringDemo { public static void main(String args[]) { Vehicle v1 = new Vehicle("Mahindra", 700); Vehicle v2 = new Vehicle("Maruti", 800); // The toString() method is called implicitly System.out.println("The value of object v1 is: " + v1); System.out.println("The value of object v2 is: " + v2); } }
Output
The output of the above code is divided into two parts. First part is the class of the object and the second part is the hexcode of the object returned by the toString() method as shown below −
The value of object v1 is: Vehicle@2c7b84de The value of object v2 is: Vehicle@3fee733d
To Continue Learning Please Login