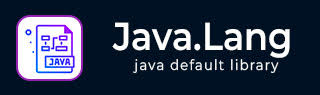
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Number min() Method
Description
The method gives the smaller of the two arguments. If the arguments have the same value, the result is that same value. If either value is NaN, then the result is NaN. Unlike the numerical comparison operators, this method considers negative zero to be strictly smaller than positive zero. If one argument is positive zero and the other is negative zero, the result is negative zero.The argument can be int, float, long, double.
Syntax
This method has the following variants −
public static double min(double arg1, double arg2) public static float min(float arg1, float arg2) public static int min(int arg1, int arg2) public static long min(long arg1, long arg2)
Parameters
Here is the detail of parameters −
- This method accepts any primitive data type as a parameter.
Return Value
- This method returns the smaller of the two arguments.
Getting Minimum of Two double Values Example
In this example, we're showing the usage of Double.min() method to get the minimum of two double values. We've created two double variables d1, d2 and initialized them with different values. Then using Double.min() method we're printing the minimum value of the given doubles.
package com.tutorialspoint; public class Test { public static void main(String args[]) { double d1 = 100.675; double d2 = 100.500; System.out.println(Double.min(d1,d2)); } }
This will produce the following result −
Output
100.5
Getting Minimum of Two float Values Example
In this example, we're showing the usage of Float.min() method to get the minimum of two float values. We've created two float variables d1, d2 and initialized them with different values. Then using Float.min() method we're printing the minimum value of the given floats.
package com.tutorialspoint; public class Test { public static void main(String args[]) { float d1 = (float)100.675; float d2 = (float)100.500; System.out.println(Float.min(d1,d2)); } }
This will produce the following result −
Output
100.5
Getting Minimum of Two int Values Example
In this example, we're showing the usage of Integer.min() method to get the minimum of two int values. We've created two int variables d1, d2 and initialized them with different values. Then using Integer.min() method we're printing the minimum value of the given ints.
package com.tutorialspoint; public class Test { public static void main(String args[]) { int d1 = 101; int d2 = 100; System.out.println(Integer.min(d1,d2)); } }
This will produce the following result −
Output
100
Getting Minimum of Two long Values Example
In this example, we're showing the usage of Long.min() method to get the minimum of two long values. We've created two long variables d1, d2 and initialized them with different values. Then using Long.min() method we're printing the minimum value of the given longs.
package com.tutorialspoint; public class Test { public static void main(String args[]) { long d1 = 101L; long d2 = 100L; System.out.println(Long.min(d1,d2)); } }
This will produce the following result −
Output
100