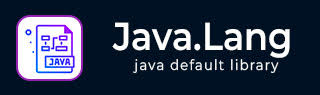
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Math.abs() Method
Description
The method gives the absolute value of the argument. The argument can be int, float, long, double, short, byte. If the argument is not negative, the argument is returned. If the argument is negative, the negation of the argument is returned.Special cases −
- If the argument is positive zero or negative zero, the result is positive zero.
- If the argument is infinite, the result is positive infinity.
- If the argument is NaN, the result is NaN.
Syntax
Following are all the variants of this method −
double abs(double d) float abs(float f) int abs(int i) long abs(long lng)
Parameters
Here is the detail of parameters −
- Any primitive data type.
Return Value
- This method Returns the absolute value of the argument.
Example 1
In this example, we're showing the usage of Math.abs() method to get absolute value of an int. We've created two Integer variable x and y and initialized them with negative and positive values. Then using Math.abs() method we're printing the absolute value of the integers.
public class Test { public static void main(String args[]) { Integer a = -8; Integer b = 8; System.out.println(Math.abs(a)); System.out.println(Math.abs(b)); } }
This will produce the following result −
Output
8 8
Example 2
In this example, we're showing the usage of Math.abs() method to get absolute value of a long. We've created two Long variable x and y and initialized them with negative and positive values. Then using Math.abs() method we're printing the absolute value of longs.
public class Test { public static void main(String args[]) { Long a = -8L; Long b = 8L; System.out.println(Math.abs(a)); System.out.println(Math.abs(b)); } }
This will produce the following result −
Output
8 8
Example 3
In this example, we're showing the usage of Math.abs() method to get absolute value of a double. We've created two Double variable x and y and initialized them with negative and positive values. Then using Math.abs() method we're printing the absolute value of the doubles.
public class Test { public static void main(String args[]) { Double a = -8.0; Double b = 8.0; System.out.println(Math.abs(a)); System.out.println(Math.abs(b)); } }
This will produce the following result −
Output
8.0 8.0