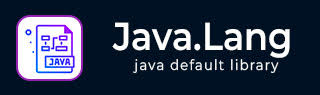
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java.lang.Class.getSigners() Method
Description
The java.lang.Class.getSigners() gets the signers of this class.
Declaration
Following is the declaration for java.lang.Class.getSigners() method
public Object[] getSigners()
Parameters
NA
Return Value
This method returns the signers of this class, or null if there are no signers and returns null if this object represents a primitive type or void.
Exception
NA
Example
The following example shows the usage of java.lang.Class.getSigners() method.
package com.tutorialspoint; import java.lang.*; public class ClassDemo { public static void main(String[] args) { try { Class cls = Class.forName("ClassDemo"); // returns the name of the class System.out.println("Class = " + cls.getName()); Object[] obj = cls.getSigners(); System.out.println("Value = " + obj); } catch(ClassNotFoundException ex) { System.out.println(ex.toString()); } } }
Let us compile and run the above program, this will produce the following result −
Class = ClassDemo Value = null
java_lang_class.htm
Advertisements
To Continue Learning Please Login