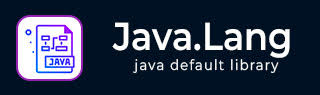
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class getResourceAsStream() Method
Description
The Java Class getResourceAsStream() method finds a resource with a given name.It returns a InputStream object or null if no resource with this name is found.
Declaration
Following is the declaration for java.lang.Class.getResourceAsStream() method
public InputStream getResourceAsStream(String name)
Parameters
name − This is the name of the desired resource.
Return Value
This method returns a InputStream object or null if no resource with this name is found.
Exception
NullPointerException − If name is null.
Getting Stream of a Resource Example
The following example shows the usage of java.lang.Class.getResourceAsStream() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved. Using getResourceAsStream(), we've retrieved the stream from a name of a file and printed the content of the same.
package com.tutorialspoint; import java.io.*; public class ClassDemo { static String getResource(String rsc) { String val = ""; try { // input stream InputStream i = ClassDemo.class.getResourceAsStream(rsc); BufferedReader r = new BufferedReader(new InputStreamReader(i)); // reads each line String l; while((l = r.readLine()) != null) { val = val + l; } i.close(); } catch(Exception e) { System.out.println(e); } return val; } public static void main(String[] args) { System.out.println("File1: " + getResource("file.txt")); System.out.println("File2: " + getResource("test.txt")); } }
Output
Assuming we have a text file file.txt, which has the following content −
This is TutorialsPoint!
Assuming we have another text file test.txt, which has the following content −
This is Java Tutorial
Let us compile and run the above program, this will produce the following result −
File1: This is TutorialsPoint! File2: This is Java Tutorial
Getting Stream of a Non-Existing Resource Example
The following example shows the usage of java.lang.Class.getResourceAsStream() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved. Using getResourceAsStream(), we've retrieved the stream from a name of a file and printed the content of the same.
package com.tutorialspoint; import java.io.*; public class ClassDemo { static String getResource(String rsc) { String val = ""; try { // input stream InputStream i = ClassDemo.class.getResourceAsStream(rsc); BufferedReader r = new BufferedReader(new InputStreamReader(i)); // reads each line String l; while((l = r.readLine()) != null) { val = val + l; } i.close(); } catch(Exception e) { System.out.println(e); } return val; } public static void main(String[] args) { System.out.println("File1: " + getResource("file1.txt")); System.out.println("File2: " + getResource("test1.txt")); } }
Output
Let us compile and run the above program, this will produce the following result −
java.lang.NullPointerException File1: java.lang.NullPointerException File2: