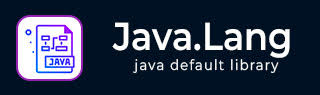
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class getInterfaces() Method
Description
The Java Class getInterfaces() method determines the interfaces implemented by the class or interface represented by this object.
Declaration
Following is the declaration for java.lang.Class.getInterfaces() method
public Class<?>[] getInterfaces()
Parameters
NA
Return Value
This method returns an array of interfaces implemented by this class.
Exception
NA
Getting Interfaces of a Class Implementing an Interface Example
The following example shows the usage of java.lang.Class.getInterfaces() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved. Using getInterfaces(), we've retrieved all interfaces implemented.
package com.tutorialspoint; import java.util.Arrays; interface Print { public void show(); } public class ClassDemo implements Print { public static void main(String[] args) { ClassDemo c = new ClassDemo(); show(c.getClass()); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } public void show(){} }
Output
Let us compile and run the above program, this will produce the following result −
Class = com.tutorialspoint.ClassDemo Classes = [] Interfaces = [interface com.tutorialspoint.Print]
Getting Interfaces of a Thread Class Example
The following example shows the usage of java.lang.Class.getInterfaces() method. In this program, we've used Thread Class and then using getInterfaces() method, we've retrieved all interfaces.
package com.tutorialspoint; import java.util.Arrays; public class ClassDemo { public static void main(String[] args) { show(Thread.class); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } }
Output
Let us compile and run the above program, this will produce the following result −
Class = java.lang.Thread Classes = [interface java.lang.Thread$UncaughtExceptionHandler, class java.lang.Thread$State] Interfaces = [interface java.lang.Runnable]
Getting Interfaces of a ArrayList Class Example
The following example shows the usage of java.lang.Class.getInterfaces() method. In this program, we've used Thread Class and then using getInterfaces() method, we've retrieved all interfaces.
package com.tutorialspoint; import java.util.Arrays; public class ClassDemo { public static void main(String[] args) { show(ArrayList.class); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } }
Output
Let us compile and run the above program, this will produce the following result −
Class = java.util.ArrayList Classes = [] Interfaces = [interface java.util.List, interface java.util.RandomAccess, interface java.lang.Cloneable, interface java.io.Serializable]