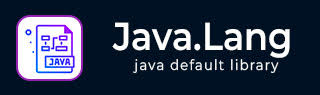
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class getEnclosingClass() Method
Description
The Java Class getEnclosingClass() method returns the immediately enclosing class of the underlying class. If this class is a top level class this method returns null.
Declaration
Following is the declaration for java.lang.Class.getEnclosingClass() method
public Class<?> getEnclosingClass()
Parameters
NA
Return Value
This method returns the immediately enclosing class of the underlying class.
Exception
NA
Getting Enclosing Class of the Underlying Class Example
The following example shows the usage of java.lang.Class.getEnclosingClass() method. In this program, we've created three classes. ClassDemo class contains two inner Classes, Outer and Inner. Outer and Inner classes have show() method which utilizes getEnclosingClass() to get the enclosing class of the underlying class. In each constructor, show method is called and result is printed.
package com.tutorialspoint; public class ClassDemo { // constructor public ClassDemo() { // class Outer as inner class for class ClassDemo class Outer { public void show() { // inner class of Class Outer class Inner { public void show() { System.out.print(getClass().getName() + " inner in..."); System.out.println(getClass().getEnclosingClass()); } } System.out.print(getClass().getName() + " inner in..."); System.out.println(getClass().getEnclosingClass()); // inner class show() function Inner i = new Inner(); i.show(); } } // outer class show() function Outer o = new Outer(); o.show(); } public static void main(String[] args) { ClassDemo cls = new ClassDemo(); } }
Output
Let us compile and run the above program, this will produce the following result −
com.tutorialspoint.ClassDemo$1Outer inner in...class com.tutorialspoint.ClassDemo com.tutorialspoint.ClassDemo$1Outer$1Inner inner in...class com.tutorialspoint.ClassDemo$1Outer
Getting Enclosing Class of the ArrayList Class Example
The following example shows the usage of java.lang.Class.getEnclosingClass() method. In this program, we've used class of ArrayList. Then using getEnclosingClass(), enclosing class is checked and printed.
package com.tutorialspoint; import java.util.ArrayList; public class ClassDemo { public static void main(String[] args) { Class cls = ArrayList.class; System.out.println(cls.getEnclosingClass()); } }
Output
Let us compile and run the above program, this will produce the following result −
null
Getting Enclosing Class of the Thread Class Example
The following example shows the usage of java.lang.Class.getEnclosingClass() method. In this program, we've used class of Thread. Then using getEnclosingClass(), enclosing class is checked and printed.
package com.tutorialspoint; public class ClassDemo { public static void main(String[] args) { Class cls = Thread.class; System.out.println(cls.getEnclosingClass()); } }
Output
Let us compile and run the above program, this will produce the following result −
null