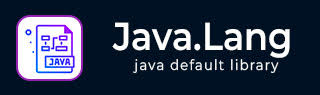
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class getDeclaredField() Method
Description
The Java Class getDeclaredField() method returns a Field object that reflects the specified declared field of the class or interface represented by this Class object. The name parameter is a String that specifies the simple name of the desired field.
Declaration
Following is the declaration for java.lang.Class.getDeclaredField() method
public Field getDeclaredField(String name) throws NoSuchFieldException, SecurityException
Parameters
name − This is the name of the field.
Return Value
This method returns the Field object for the specified field in this class.
Exception
NoSuchFieldException − If a field with the specified name is not found.
NullPointerException − If name is null.
SecurityException − If a security manager, s, is present.
Getting Declared Field of a Class Example
The following example shows the usage of java.lang.Class.getDeclaredField() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved. Using getDeclaredField(), we've retrieved the required Field and printed it.
package com.tutorialspoint; import java.lang.reflect.Field; public class ClassDemo { public static void main(String[] args) { try { ClassDemo c = new ClassDemo(); Class cls = c.getClass(); // field long l Field lVal = cls.getDeclaredField("l"); System.out.println("Field = " + lVal.toString()); } catch(Exception e) { System.out.println(e.toString()); } } public ClassDemo() { // no argument constructor } public ClassDemo(long l) { this.l = l; } long l = 77688; }
Output
Let us compile and run the above program, this will produce the following result −
Field = long com.tutorialspoint.ClassDemo.l
Getting Declared Field of an ArrayList Example
The following example shows the usage of java.lang.Class.getDeclaredField() method. In this program, we've used class of ArrayList. Using getDeclaredField(), we've retrieved the required Field and printed it.
package com.tutorialspoint; import java.lang.reflect.Field; import java.util.ArrayList; public class ClassDemo { public static void main(String[] args) { try { Class cls = ArrayList.class; // field int size Field size = cls.getDeclaredField("size"); System.out.println("Field = " + size.toString()); } catch(Exception e) { System.out.println(e.toString()); } } }
Output
Let us compile and run the above program, this will produce the following result −
Field = private int java.util.ArrayList.size
Getting Declared Field of a Thread Example
The following example shows the usage of java.lang.Class.getDeclaredField() method. In this program, we've used class of Thread. Using getDeclaredField(), we've retrieved the required Field and printed it.
package com.tutorialspoint; import java.lang.reflect.Field; public class ClassDemo { public static void main(String[] args) { try { Class cls = Thread.class; // field long tid Field tid = cls.getDeclaredField("tid"); System.out.println("Field = " + tid.toString()); } catch(Exception e) { System.out.println(e.toString()); } } }
Output
Let us compile and run the above program, this will produce the following result −
Field = private final long java.lang.Thread.tid