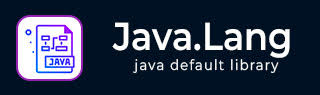
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class getConstructors() Method
Description
The Java Class getConstructors() method returns an array containing Constructor objects reflecting all the public constructors of the class represented by this Class object. An array of length 0 is returned if the class has no public constructors, or if the class is an array class, or if the class reflects a primitive type or void.
Declaration
Following is the declaration for java.lang.Class.getConstructors() method
public Constructor<?>[] getConstructors() throws SecurityException
Parameters
NA
Return Value
This method returns the array of Constructor objects representing the public constructors of this class.
Exception
SecurityException − If a security manager, s, is present.
Getting Constructors of a Class Example
The following example shows the usage of java.lang.Class.getConstructors() method. In this program, we've created an instance of Class for java.awt.Panel class. Now using getConstructors() method, the constructors of the class are retrieved and the result is printed.
package com.tutorialspoint; import java.lang.reflect.Constructor; public class ClassDemo { public static void main(String[] args) { try { Class cls = Class.forName("java.awt.Panel"); System.out.println("Panel Constructors ="); /* returns the array of Constructor objects representing the public constructors of this class */ Constructor constructors[] = cls.getConstructors(); for(int i = 0; i < constructors.length; i++) { System.out.println(constructors[i]); } } catch (Exception e) { System.out.println("Exception: " + e); } } }
Output
Let us compile and run the above program, this will produce the following result −
Panel Constructors = public java.awt.Panel() public java.awt.Panel(java.awt.LayoutManager)
Getting Constructors of a Custom Class Example
The following example shows the usage of java.lang.Class.getConstructors() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved. Now using getConstructors() method, the constructors of the class are retrieved and the result is printed.
package com.tutorialspoint; import java.lang.reflect.Constructor; public class ClassDemo { public static void main(String[] args) { try { ClassDemo c = new ClassDemo(); Class cls = c.getClass(); System.out.print("Class Constructors = "); /* returns the array of Constructor objects representing the public constructors of this class */ Constructor[] constructors = cls.getConstructors(); for(int i = 0; i < constructors.length; i++) { System.out.println(constructors[i]); } } catch (Exception e) { System.out.println("Exception: " + e); } } }
Output
Let us compile and run the above program, this will produce the following result −
Class Constructors = public com.tutorialspoint.ClassDemo()
Getting Constructors of ArrayList Class Example
The following example shows the usage of java.lang.Class.getConstructors() method. In this program, we've retrieved ArrayList class. Now using getConstructors() method, the constructors of the class are retrieved and the result is printed.
package com.tutorialspoint; import java.lang.reflect.Constructor; import java.util.ArrayList; public class ClassDemo { public static void main(String[] args) { try { Class cls = ArrayList.class; System.out.println("List Constructors ="); /* returns the array of Constructor objects representing the public constructors of this class */ Constructor constructors[] = cls.getConstructors(); for(int i = 0; i < constructors.length; i++) { System.out.println(constructors[i]); } } catch (Exception e) { System.out.println("Exception: " + e); } } }
Output
Let us compile and run the above program, this will produce the following result −
List Constructors = public java.util.ArrayList(java.util.Collection) public java.util.ArrayList() public java.util.ArrayList(int)