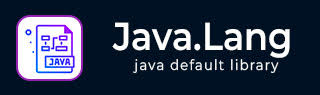
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Boolean valueOf(boolean) Method
Description
The Java Boolean valueOf(boolean b) returns a Boolean instance representing the specified boolean value. If the specified boolean value is true, this method returns Boolean.TRUE; if it is false, this method returns Boolean.FALSE.
If a new Boolean instance is not required, this method should generally be used in preference to the constructor Boolean(boolean), as this method is likely to yield significantly better space and time performance.
Declaration
Following is the declaration for java.lang.Boolean.valueOf() method
public static Boolean valueOf(boolean b)
Parameters
b − a boolean value
Return Value
This method returns a Boolean instance representing b.
Exception
NA
Getting Boolean from a boolean primitive as true Example
The following example shows the usage of Boolean valueOf() method for a boolean value as true. In this program, we've created a Boolean variable an using valueOf() method, we've retrieved a Boolean object with underlying value as false from a boolean true. Then result is printed.
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 1 Boolean object b1 Boolean b1; // create 1 boolean primitive and assign value boolean bool1 = true; /** * static method is called using class name * assign result of valueOf method on bool1 to b1 */ b1 = Boolean.valueOf(bool1); String str1 = "Boolean instance of primitive " + bool1 + " is " + b1; // print b1 values System.out.println( str1 ); } }
Output
Let us compile and run the above program, this will produce the following result −
Boolean instance of primitive true is true
Getting Boolean from a boolean primitive as false Example
The following example shows the usage of Boolean valueOf() method for a boolean value as false. In this program, we've created a Boolean variable an using valueOf() method, we've retrieved a Boolean object with underlying value as false from a boolean false. Then result is printed.
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 1 Boolean object b1 Boolean b1; // create 1 boolean primitive and assign value boolean bool1 = false; /** * static method is called using class name * assign result of valueOf method on bool1 to b1 */ b1 = Boolean.valueOf(bool1); String str1 = "Boolean instance of primitive " + bool1 + " is " + b1; // print b1 values System.out.println( str1 ); } }
Output
Let us compile and run the above program, this will produce the following result −
Boolean instance of primitive false is false