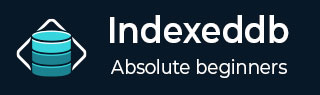
- IndexedDB Tutorial
- IndexedDB - Home
- IndexedDB - Introduction
- IndexedDB - Installation
- IndexedDB - Connection
- IndexedDB - Object Stores
- IndexedDB - Creating Data
- IndexedDB - Reading Data
- IndexedDB - Updating Data
- IndexedDB - Deleting Data
- Using getAll() Functions
- IndexedDB - Indexes
- IndexedDB - Ranges
- IndexedDB - Transactions
- IndexedDB - Error Handling
- IndexedDB - Searching
- IndexedDB - Cursors
- IndexedDB - Promise Wrapper
- IndexedDB - Ecmascript Binding
- IndexedDB Useful Resources
- IndexedDB - Quick Guide
- IndexedDB - Useful Resources
- IndexedDB - Discussion
IndexedDB - Transactions
A transaction is a group of operations, that should either all succeed, or all fail. For example, if we pay from UPI to a merchant and the transaction declines then the money must fall back to the senders' account. A transaction maintains this integrity.
Following is the syntax for opening a transaction −
db.transaction(store[, type]);
The store here is the object store where we want to do the transaction. The type of the transaction is of 2 types −
read-only − can only read, by default it is given.
read-write − we can only read and write the data but we cannot create, remove or alter it from object stores.
Transaction life cycle
The transaction is a connection between object stores to perform any operations. Each transaction has a state which can be −
active − When a transaction is first created. or when a request is associated with a transaction. New requests can be made against the transaction when it is in this state.
inactive − A transaction is in this state after control returns to the event after its creation. No requests can be made against the transaction when it is in this state.
committing − Once all the requests associated with a transaction are completed then it attempts to commit. During the commit state, no new requests can be made.
finished − After a transaction is committed or aborted then it is in the finished state. During the finished state, no new requests can be made.
The lifetime of a transaction
A transaction with a scope and a mode is formed. A transaction's state is originally active when it is generated.
To begin the transaction, the implementation must queue a task.
A success or, error event will be fired when each request connected with a transaction is handled. The transaction state is set to active when the event is sent, allowing subsequent requests to be made against the transaction. The transaction's status is set to inactive once the event dispatch is completed.
A transaction can be canceled at any point before it is completed, even if it isn't currently active or hasn't begun.
When all requests made against the database are successful, the implementation must attempt to commit the transaction.
When a transaction is committed or aborted its state is set to finish.
Transaction scheduling
When a transaction can be started, there are some limitations.
When there is no read or write transactions that: a. were established before transactions tx b. have an overlapping scope with tx c. are not in a final state, a read-only transaction tx can commence.
A read/write-only transaction tx can begin when there are no other transactions that −
- were formed before tx,
- have an overlapping scope with tx, or
- are not in a completed state.
Upgrade transactions
A transaction with the mode "versionchange" is an upgrade transaction.
Object stores and indexes in a database can be created, renamed, and deleted using upgrade operations.
If a version greater than the current version is given, an upgrade transaction is automatically produced when completing the steps to run an upgrade transaction after a connection to a database is opened. Within the upgradeneeded event handler, this transaction will be active.
Committing a transaction
The following steps must be completed to commit a transaction −
- The transaction state is first set to committing.
- Wait until all of the transaction requests on the list have been processed.
- Abort the transaction if an error occurs.
- If the transaction is an upgrade transaction, set the database upgrade transaction's transactions connections to NULL.
- Change the transaction state to complete.
- fire the transaction's entire event
- If a transaction is an upgrade transaction, set the request's transaction to null and make the request that is associated with the transaction.
Syntax
transaction.commit()
Attempts to commit the transaction. All pending requests will be allowed to complete, but no new requests will be accepted.
The transaction will abort if a pending request fails. The success events for successful requests will still fire, but throwing an exception in an event handler will not abort the transaction calling preventDefault() will not prevent the transaction from aborting.
Event Handlers
Various event handler attributes are as follows
attribute EventHandler onabort; attribute EventHandler oncomplete; attribute EventHandler onerror;
Transactions Example
A simple JavaScript program to demonstrate the usage of a transaction is given below −
<!DOCTYPE html> <html lang="en"> <head> <title>Document</title> </head> <body> <script> const request = indexedDB.open("botdatabase",1); request.onupgradeneeded = function(){ const db = request.result; const store = db.createObjectStore("bots",{ keyPath: "id"}); } request.onsuccess = function(){ document.write("database opened successfully"); const db = request.result; const transaction=db.transaction("bots","readwrite"); const store = transaction.objectStore("bots"); store.add({id: 1, name: "jason",branch: "IT"}); store.add({id: 2, name: "praneeth",branch: "CSE"}); store.add({id: 3, name: "palli",branch: "EEE"}); store.add({id: 4, name: "abdul",branch: "IT"}); store.put({id: 4, name: "deevana",branch: "CSE"}); transaction.oncomplete = function(){ document.write("transaction complete"); db.close; }; } </script> </body> </html>
Output
database opened successfully transaction complete
Here a transaction is created, only when a transaction is created can we add data into the object store and finally after the transaction is complete we close the database.
Example
The following example shows the usage of a transaction attribute oncomplete −
function student(db, names) { let transaction = db.transaction(['names'], 'readwrite'); let store = transaction.objectStore('names'); for (let i = 0; i < messages.length; i++) { store.add({text: names[i]}); } transaction.oncomplete = function() {document.write('transaction complete')}; }
Abort transactions
To abort a transaction, follow these steps −
All transaction-related modifications to the database are undone.
Changes in object stores, indexes, and versions are likewise reverted during upgrade transactions.
Complete the transaction state.
Set transactions error to error if the error is not null.
Set the request processed flag to true for each request in the transactions request list.
Set the requests done flag to true and the results to define.
If the transaction is an upgrade transaction, set the upgrade transaction associated with the transaction's connection to null.
Create an abort at transaction event with the bubbles attribute set to true.
If the transaction is an upgrade transaction, assume that request is the transaction's open request.
To Continue Learning Please Login