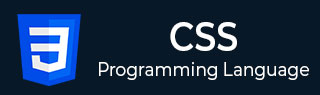
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Data Type - <time>
The CSS <time> data type represents a duration of time. It is used in properties where a time value is expected, such as animation-duration, transition-duration, and certain other properties. The value for <time> can be specified in seconds (s), milliseconds (ms), or other time units.
Possible Values
Following units can be used with the <time> data type:
s - Denotes a time interval expressed in seconds.
ms - Denotes a time interval expressed in milliseconds.
Syntax
<number>unit
The <number> in the <time> data type is followed by the particular units mentioned above. Either a single + or - sign may optionally precede it.
The unit literal and the number should not be separated, just like in other dimensions.
CSS <time> - Valid Syntax
Following is the list of valid times:
Time | Description |
---|---|
19.6 | Positive integer |
-123ms | Negative integer. |
2.6ms | Non-integer |
10mS | Although it's not necessary to use capital letters, the unit is case-insensitive. |
+0s | Zero with a unit and a leading + |
-0ms | Zero, a unit, and a leading - |
CSS <time> - InValid Syntax
Following is the list of invalid times:
Time | Description |
---|---|
0 | Unitless zero is not valid for <time>s, however it is allowed for <length>s. |
14.0 | This lacks a unit, therefore it's a <number> rather than a <time>. |
9 ms | No space is allowed between the number and the unit. |
CSS <time> - Valid/Invalid Time Check
The following example allows you to check the provided input is valid or invalid time
<html> <head> <style> body { font-family: Arial, sans-serif; } .container { width: 50%; margin: 50px auto; text-align: center; } label { margin-right: 10px; } input { padding: 5px; margin-right: 10px; } button { padding: 5px 10px; cursor: pointer; } #result { margin-top: 20px; font-weight: bold; } </style> </head> <body> <div class="container"> <h2>Time Input Validation</h2> <form id="timeForm"> <label for="timeInput">Enter a time:</label> <input type="text" id="timeInput" name="timeInput" placeholder="e.g., 5s or -200ms or 10mS"> <button type="button" onclick="validateTime()">Check</button> </form> <p id="result"></p> </div> <script> function validateTime() { const userInput = document.getElementById('timeInput').value.trim(); // Regular expressions to match valid time inputs const validTimeRegex = /^(0|(\+|-)?\d+(\.\d+)?(s|ms))$/i; if (validTimeRegex.test(userInput)) { document.getElementById('result').innerText = 'Valid time input!'; } else { document.getElementById('result').innerText = 'Invalid time input!'; } } </script> </body> </html>
CSS <time> - Used With transition-duration
When used within transition-duration in CSS, the <time> data type defines a duration for a transition effect, indicating how long the transition will take to finish.
It enables for exact control over the time of CSS transitions and may be defined in milliseconds or seconds.
<html> <head> <style> button { transition-property: background-color, color, transform; transition-duration: 3s; transition-timing-function: cubic-bezier(0.25, 0.46, 0.45, 0.94); background-color: #2ecc71; color: white; border: none; padding: 15px 30px; font-size: 18px; cursor: pointer; border-radius: 8px; outline: none; box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1); } button:hover { background-color: #3498db; color: #fff; transform: translateY(-3px); box-shadow: 0 6px 8px rgba(0, 0, 0, 0.15); } </style> </head> <body> <button>Hover Over This Button for 3 seconds</button> </body> </html>