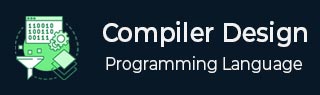
- Compiler Design Tutorial
- Compiler Design - Home
- Compiler Design - Overview
- Compiler Design - Architecture
- Phases
- Compiler Design - Phases
- Compiler Design - Global Optimization
- Compiler Design - Local Optimization
- Lexical Analysis
- Compiler Design - Lexical Analysis
- Compiler Design - Regular Expressions
- Compiler Design - Finite Automata
- Compiler Design - Language Elements
- Compiler Design - Lexical Tokens
- Compiler Design - FSM
- Compiler Design - Lexical Table
- Compiler Design - Sequential Search
- Compiler Design - Binary Search Tree
- Compiler Design - Hash Table
- Syntax Analysis
- Compiler Design - Syntax Analysis
- Compiler Design - Parsing Types
- Compiler Design - Grammars
- Compiler Design - Classes Grammars
- Compiler Design - Pushdown Automata
- Compiler Design - Ambiguous Grammar
- Parsing
- Compiler Design - Top-Down Parser
- Compiler Design - Bottom-Up Parser
- Compiler Design - Simple Grammar
- Compiler Design - Quasi-Simple Grammar
- Compiler Design - LL(1) Grammar
- Error Recovery
- Compiler Design - Error Recovery
- Semantic Analysis
- Compiler Design - Semantic Analysis
- Compiler Design - Symbol Table
- Run Time
- Compiler Design - Run-time Environment
- Code Generation
- Compiler Design - Code Generation
- Converting Atoms to Instructions
- Compiler Design - Transfer of Control
- Compiler Design - Register Allocation
- Forward Transfer of Control
- Reverse Transfer of Control
- Code Optimization
- Compiler Design - Code Optimization
- Compiler Design - Intermediate Code
- Basic Blocks and DAGs
- Control Flow Graph
- Compiler Design - Peephole Optimization
- Implementing Translation Grammars
- Compiler Design - Attributed Grammars
- Compiler Design Resources
- Compiler Design - Quick Guide
- Compiler Design - Useful Resources
- Compiler Design - Discussion
Compiler Design - Peephole Optimization
Peephole optimization is a method used to optimize a small set of compiler-generated instructions; this small set is referred to as the peephole optimization or window in compiler design.
Key Points of Peephole Optimization
Key points about peephole optimization
The key points about peephole optimization are listed below −
- It is applied after the source code is converted into the target code.
- Peephole optimization falls under machine-dependent optimization. Machine-dependent optimization takes place after the target code is generated and adapted to the machine's architecture. It uses CPU registers and may use absolute memory references instead of relative ones.
- It is applied repeatedly to small sections of code.
Goals of Peephole Optimization
The goals of peephole optimization include −
- Improving performance
- Reducing memory footprint
- Reducing code size.
Working of Peephole Optimization in Compiler Design
The working of Peephole optimization in compiler design can be summarized in the following steps −
Step 1: Identify the Peephole
In the first step, the compiler finds the small sections of the generated code that need optimization. Peephole refers to an instruction within a fixed window size, so the window size depends on the specific optimization being performed. The compiler helps to define the instructions within the window.
Step 2: Apply the Optimization Rule
After identification, in the second step, the compiler applies a predefined set of optimization rules to the instructions in the peephole. The compiler will search for the specific pattern of instructions in the window. There can be many types of patterns, such as insufficient code, series of loads and stores, or complex patterns like branches.
Step 3: Evaluate the Result
After the pattern is identified, the compiler makes the changes in the instructions. The compiler then cross-checks the code to determine if the changes improved the code. It checks the improvement based on size, speed, and memory usage.
Step 4: Repeat
The above steps will go on in a loop by finding the peephole repeatedly until no more optimization is left in the code. The compiler will go to each instruction one at a time, make the changes, and reanalyze it for the best result.
Objectives of Peephole Optimization in Compiler Design
The following are the objectives of peephole optimization in compiler design −
- Increasing code speed − Peephole optimization aims to enhance the execution speed of the generated code by removing redundant or unnecessary instructions.
- Reduced code size: − Peephole optimization works to reduce the size of the generated code by replacing long sequences of instructions with shorter ones.
- Getting rid of dead code − Peephole optimization targets the removal of dead code, such as unreachable code, redundant assignments, or constant expressions that have no impact on the program's output.
- Simplifying code − Peephole optimization aims to make the generated code more understandable and manageable by eliminating unnecessary complexities.
Peephole Optimization Techniques
The following are the techniques of peephole optimization −
Constant Folding
Constant folding is a peephole optimization technique that involves evaluating constant expressions during compile-time rather than at run-time. This can improve performance by reducing the number of computations needed during execution.
Example
The following is an example of a constant folding technique −
Initial Code
int x = 10 + 5; int y = x * 2;
Optimized Code
int x = 15; int y = x * 2;
Strength Reduction
Strength reduction is a peephole optimization technique that replaces computationally expensive operations with simpler, cheaper ones, enhancing program performance.
Example
The following is an example of a constant folding technique −
Initial Code
int x = y / 4;
Optimized Code
int x = y >> 2;
Redundant Load and Store Elimination
Redundant load and store elimination is a peephole optimization technique that reduces unnecessary memory accesses in a program. It eliminates repeated memory operations by reusing already loaded values.
Initial Code
int x = 5; int y = x + 10; int z = x + 20;
Optimized Code
int x = 5; int y = x + 10; int z = y + 10;
Null Sequences Elimination
Null sequence elimination is a peephole optimization technique that removes unnecessary instructions from a program. It identifies and eliminates sequences of instructions that do not affect the final output of the program.
Example
The following is an example of a constant folding technique −
Initial Code
int x = 5; int y = 10; int z = x + y; x = 5; // redundant instruction
Optimized Code
int x = 5; int y = 10; int z = x + y;