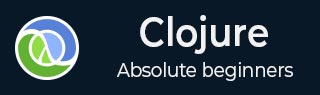
- Clojure Tutorial
- Clojure - Home
- Clojure - Overview
- Clojure - Environment
- Clojure - Basic Syntax
- Clojure - REPL
- Clojure - Data Types
- Clojure - Variables
- Clojure - Operators
- Clojure - Loops
- Clojure - Decision Making
- Clojure - Functions
- Clojure - Numbers
- Clojure - Recursion
- Clojure - File I/O
- Clojure - Strings
- Clojure - Lists
- Clojure - Sets
- Clojure - Vectors
- Clojure - Maps
- Clojure - Namespaces
- Clojure - Exception Handling
- Clojure - Sequences
- Clojure - Regular Expressions
- Clojure - Predicates
- Clojure - Destructuring
- Clojure - Date & Time
- Clojure - Atoms
- Clojure - Metadata
- Clojure - StructMaps
- Clojure - Agents
- Clojure - Watchers
- Clojure - Macros
- Clojure - Reference Values
- Clojure - Databases
- Clojure - Java Interface
- Clojure - Concurrent Programming
- Clojure - Applications
- Clojure - Automated Testing
- Clojure - Libraries
- Clojure Useful Resources
- Clojure - Quick Guide
- Clojure - Useful Resources
- Clojure - Discussion
Clojure - Strings
A String literal is constructed in Clojure by enclosing the string text in quotations. Strings in Clojure need to be constructed using the double quotation marks such as “Hello World”.
Example
Following is an example of the usage of strings in Clojure.
(ns clojure.examples.hello (:gen-class)) (defn hello-world [] (println "Hello World") (println "This is a demo application")) (hello-world)
Output
The above program produces the following output.
Hello World This is a demo application
Basic String Operations
Clojure has a number of operations that can be performed on strings. Following are the operations.
Sr.No. | String Operations & Description |
---|---|
1 | str
The concatenation of strings can be done by the simple str function. |
2 | format
The formatting of strings can be done by the simple format function. The format function formats a string using java.lang.String.format. |
3 | count
Returns the number of characters in the string. |
4 | subs
Returns the substring of ‘s’ beginning at start inclusive, and ending at end (defaults to length of string), exclusive. |
5 | compare
Returns a negative number, zero, or a positive number when ‘x’ is logically 'less than', 'equal to', or 'greater than' ‘y’. |
6 | lower-case
Converts string to all lower-case. |
7 | upper-case
Converts string to all upper-case. |
8 | join
Returns a string of all elements in collection, as returned by (seq collection), separated by an optional separator. |
9 | split
Splits string on a regular expression. |
10 | split-lines
Split strings is based on the escape characters \n or \r\n. |
11 | reverse
Reverses the characters in a string. |
12 | replace
Replaces all instance of a match in a string with the replacement string. |
13 | trim
Removes whitespace from both ends of the string. |
14 | triml
Removes whitespace from the left hand side of the string. |
15 | trimr
Removes whitespace from the right hand side of the string. |