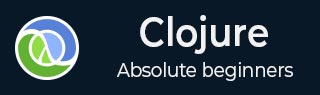
- Clojure Tutorial
- Clojure - Home
- Clojure - Overview
- Clojure - Environment
- Clojure - Basic Syntax
- Clojure - REPL
- Clojure - Data Types
- Clojure - Variables
- Clojure - Operators
- Clojure - Loops
- Clojure - Decision Making
- Clojure - Functions
- Clojure - Numbers
- Clojure - Recursion
- Clojure - File I/O
- Clojure - Strings
- Clojure - Lists
- Clojure - Sets
- Clojure - Vectors
- Clojure - Maps
- Clojure - Namespaces
- Clojure - Exception Handling
- Clojure - Sequences
- Clojure - Regular Expressions
- Clojure - Predicates
- Clojure - Destructuring
- Clojure - Date & Time
- Clojure - Atoms
- Clojure - Metadata
- Clojure - StructMaps
- Clojure - Agents
- Clojure - Watchers
- Clojure - Macros
- Clojure - Reference Values
- Clojure - Databases
- Clojure - Java Interface
- Clojure - Concurrent Programming
- Clojure - Applications
- Clojure - Automated Testing
- Clojure - Libraries
- Clojure Useful Resources
- Clojure - Quick Guide
- Clojure - Useful Resources
- Clojure - Discussion
Clojure - Sequences
Sequences are created with the help of the ‘seq’ command. Following is a simple example of a sequence creation.
(ns clojure.examples.example (:gen-class)) ;; This program displays Hello World (defn Example [] (println (seq [1 2 3]))) (Example)
The above program produces the following output.
(1 2 3)
Following are the various methods available for sequences.
Sr.No. | Methods & Description |
---|---|
1 | cons
Returns a new sequence where ‘x’ is the first element and ‘seq’ is the rest. |
2 | conj
Returns a new sequence where ‘x’ is the element that is added to the end of the sequence. |
3 | concat
This is used to concat two sequences together. |
4 | distinct
Used to only ensure that distinct elements are added to the sequence. |
5 | reverse
Reverses the elements in the sequence. |
6 | first
Returns the first element of the sequence. |
7 | last
Returns the last element of the sequence. |
8 | rest
Returns the entire sequence except for the first element. |
9 | sort
Returns a sorted sequence of elements. |
10 | drop
Drops elements from a sequence based on the number of elements, which needs to be removed. |
11 | take-last
Takes the last list of elements from the sequence. |
12 | take
Takes the first list of elements from the sequence. |
13 | split-at
Splits the sequence of items into two parts. A location is specified at which the split should happen. |