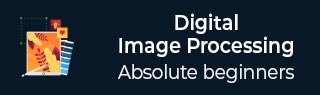
- Java Digital Image Processing
- DIP - Home
- DIP - Introduction
- DIP - Java BufferedImage Class
- DIP - Image Download & Upload
- DIP - Image Pixels
- DIP - Grayscale Conversion
- DIP - Enhancing Image Contrast
- DIP - Enhancing Image Brightness
- DIP - Enhancing Image Sharpness
- DIP - Image Compression Technique
- DIP - Adding Image Border
- DIP - Image Pyramids
- DIP - Basic Thresholding
- DIP - Image Shape Conversions
- DIP - Gaussian Filter
- DIP - Box Filter
- DIP - Eroding & Dilation
- DIP - Watermark
- DIP - Understanding Convolution
- DIP - Prewitt Operator
- DIP - Sobel Operator
- DIP - Kirsch Operator
- DIP - Robinson Operator
- DIP - Laplacian Operator
- DIP - Weighted Average Filter
- DIP - Create Zooming Effect
- DIP - Open Source Libraries
- DIP - Introduction To OpenCV
- DIP - GrayScale Conversion OpenCV
- DIP - Color Space Conversion
- DIP Useful Resources
- DIP - Quick Guide
- DIP - Useful Resources
- DIP - Discussion
Java DIP - Image Compression Technique
An image can easily be compressed and stored through Java. Compression of image involves converting an image into jpg and storing it.
In order to compress an image, we read the image and convert into BufferedImage object.
Further, we get an ImageWriter from getImageWritersByFormatName() method found in the ImageIO class. From this ImageWriter, create an ImageWriteParam object. Its syntax is given below −
Iterator<ImageWriter> list = ImageIO.getImageWritersByFormatName("jpg"); ImageWriteParam obj = writer_From_List.getDefaultWriteParam();
From this ImageWriteParam object, you can set the compression by calling these two methods which are setCompressionMode() and setCompressionQuality(). Their syntaxes are as given below −
obj.setCompressionMode(ImageWriteParam.MODE_EXPLICIT); obj.setCompressionQuality(0.05f);
The setCompressionMode() method takes Mode_EXPLICIT as the parameter. Some of the other MODES are described briefly −
Sr.No. | Modes |
---|---|
1 |
MODE_DEFAULT It is a constant value that may be passed into methods to enable that feature for future writes. |
2 |
MODE_DISABLED It is a constant value that may be passed into methods to disable that feature for future writes. |
3 |
MODE_EXPLICIT It is a constant value that may be passed into methods to enable that feature for future writes. |
Apart from the compressions methods, there are other methods provided by the ImageWriteParam class. They are described briefly −
Sr.No. | Method & Description |
---|---|
1 |
canOffsetTiles() It returns true if the writer can perform tiling with non-zero grid offsets while writing. |
2 |
getBitRate(float quality) It returns a float indicating an estimate of the number of bits of output data for each bit of input image data at the given quality level. |
3 |
getLocale() It returns the currently set Locale, or null if only a default Locale is supported. |
4 |
isCompressionLossless() It returns true if the current compression type provides lossless compression. |
5 |
unsetCompression() It removes any previous compression type and quality settings. |
6 |
unsetTiling() It removes any previous tile grid parameters specified by calls to setTiling. |
Example
The following example demonstrates the use of ImageWriteParam class to compress an image −
import java.io.*; import java.util.*; import java.awt.image.*; import javax.imageio.*; import javax.imageio.stream.ImageOutputStream; class Compression { public static void main(String[] args) throws IOException { File input = new File("digital_image_processing.jpg"); BufferedImage image = ImageIO.read(input); File compressedImageFile = new File("compress.jpg"); OutputStream os =new FileOutputStream(compressedImageFile); Iterator<ImageWriter>writers = ImageIO.getImageWritersByFormatName("jpg"); ImageWriter writer = (ImageWriter) writers.next(); ImageOutputStream ios = ImageIO.createImageOutputStream(os); writer.setOutput(ios); ImageWriteParam param = writer.getDefaultWriteParam(); param.setCompressionMode(ImageWriteParam.MODE_EXPLICIT); param.setCompressionQuality(0.05f); writer.write(null, new IIOImage(image, null, null), param); os.close(); ios.close(); writer.dispose(); } }
Output
When you execute the given code, it compresses the image digital_image_processing.jpg to its equivalent compressed image and writes it on the hard disk with the name compress.jpg.
Original Image
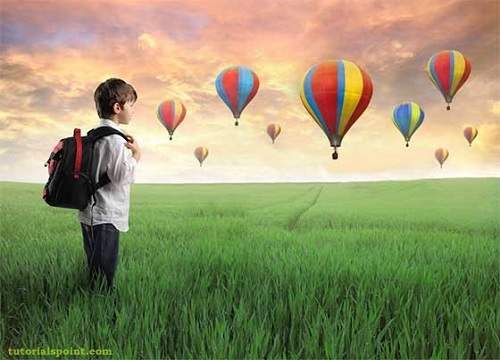
Compressed Image - Quality Factor − 0.05
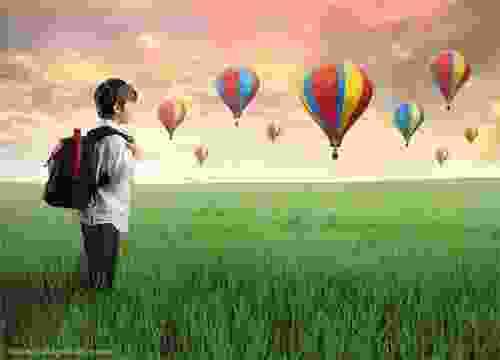
Compressed Image - Quality Factor − 0.5
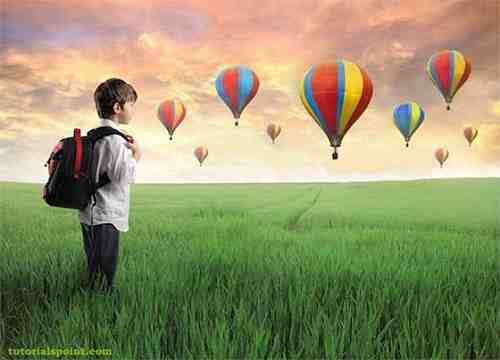