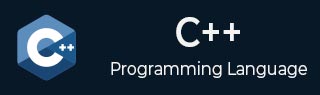
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C++ Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
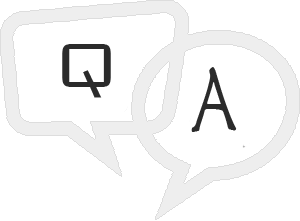
Q 1 - What is the output of the following program?
#include<iostream> using namespace std; class abc { public: static int x; int i; abc() { i = ++x; } }; int abc::x; main() { abc m, n, p; cout<<m.x<<" "<<m.i<<endl; }
Answer : A
Explaination
The static member variable ‘x’ shares common memory among all the objects created for the class.
#include<iostream> using namespace std; class abc { public: static int x; int i; abc() { i = ++x; } }; int abc::x; main() { abc m, n, p; cout<<m.x<<" "<<m.i<<endl; }
Q 2 - Choose the respective delete operator usage for the expression ‘ptr=new int[100]’.
Answer : C
Explaination
Q 3 - Runtime polymorphism is done using.
Answer : C
Explaination
Virtual functions gives the ability to override the functionality of base class into the derived class. Hence achieving dynamic/runtime polymorphism.
Q 4 - How many number of arguments can a destructor of a class receives?
Answer : A
Explaination
The destructor receives no arguments and is only form to be provided. Hence destructor cannot be overloaded.
Q 5 - What is the output of the following program?
#include<iostream> using namespace std; main() { int const a = 5; a++; cout<<a; }
Answer : D
Explaination
Compile error - constant variable cannot be modified.
Q 6 - HAS-A relationship between the classes is shown through.
Answer : B
Explaination
A class containing anther class object as its member is called as container class and exhibits HAS A relationship.
Q 7 - An exception is __
Answer : A
Explaination
When the program is in execution phase the possible unavoidable error is called as an exception.
Answer : D
Explaination
The size of ‘int’ depends upon the complier i.e. whether it is a 16 bit or 32 bit.
Q 9 - What is the output of the following program?
#include<iostream> using namespace std; int x = 5; int &f() { return x; } main() { f() = 10; cout<<x; }
Answer : D
Explaination
A function can return reference, hence it can appear on the left hand side of the assignment operator.
#include<iostream> using namespace std; int x = 5; int &f() { return x; } main() { f() = 10; cout<<x; }
Q 10 - What is the output of the following program?
#include<iostream> using namespace std; void main() { char *s = "C++"; cout<<s<<" "; s++; cout<<s<<" "; }
Answer : B
Explaination
After s++, s points the string “++”.
#include<iostream> using namespace std; void main() { char *s = "C++"; cout<<s<<" "; s++; cout<<s<<" "; }
To Continue Learning Please Login