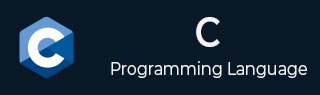
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
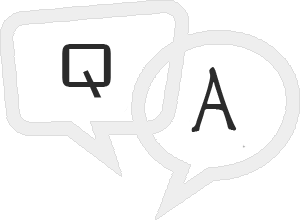
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { for()printf("Hello"); }
Answer : D
Explanation
Compiler error, semi colons need to appear though the expressions are optional for the ‘for’ loop.
Q 2 - Which operator is used to continue the definition of macro in the next line?
Answer : D
Explanation
\, the first two are stringize and token pasting operators respectively. There is no such operator called $.
Answer : B
Explanation
‘cc’ full form is C Compiler and is the compiler for UNIX. gcc is GNU C compiler for linux. Borland and vc++ (Microsoft visual c++) for windows.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { fprintf(stdout,"Hello, World!"); }
Answer : C
Explanation
stdout is the identifier declared in the header file stdio.h, need to include the same.
Q 5 - What is the output of the following program?
#include<stdio.h> main() { int x; float y; y = x = 7.5; printf("x=%d y=%f", x, y); }
Answer : A
Explanation
‘x’ gets the integral value from 7.5 which is 7 and the same is initialized to ‘y’.
Q 6 - Choose the correct function which can return a reminder by dividing -10.0/3.0?
Answer : B
Explanation
In the C Programming Language, the fmod() function compute and returns the floating-point remainder when x is divided by y.
#include <math.h> #include <stdio.h> int main( void ) { double x = -10.0, y = 3.0, z; z = fmod( x, y ); printf( "The remainder of %.2f / %.2f is %f\n", w, x, z ); }
Q 7 - Which library function can convert an integer/long to a string?
Answer : A
Explanation
In C, ltoa() function converts long/integer data type to string data type.
char *ltoa(long N, char *str, int base);
Answer : B
Explanation
Two immediate ampersand (&) symbols is logical AND operator.
Q 9 - Choose the correct order from given below options for the calling function of the code “a = f1(23, 14) * f2(12/4) + f3();”?
Answer : D
Explanation
Evaluation order is implementation dependent.
Q 10 - In the given below code, what will be the value of a variable x?
#include<stdio.h> int main() { int y = 100; const int x = y; printf("%d\n", x); return 0; }
Answer : A
Explanation
Although, integer y = 100; and constant integer x is equal to y. here in the given above program we have to print the x value, so that it will be 100.
To Continue Learning Please Login