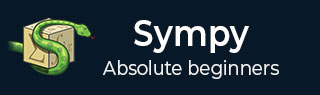
- SymPy Tutorial
- SymPy - Home
- SymPy - Introduction
- SymPy - Installation
- SymPy - Symbolic Computation
- SymPy - Numbers
- SymPy - Symbols
- SymPy - Substitution
- SymPy - sympify() function
- SymPy - evalf() function
- SymPy - Lambdify() function
- SymPy - Logical Expressions
- SymPy - Querying
- SymPy - Simplification
- SymPy - Derivative
- SymPy - Integration
- SymPy - Matrices
- SymPy - Function class
- SymPy - Quaternion
- SymPy - Solvers
- SymPy - Plotting
- SymPy - Entities
- SymPy - Sets
- SymPy - Printing
- SymPy Useful Resources
- SymPy - Quick Guide
- SymPy - Useful Resources
- SymPy - Discussion
SymPy - Solvers
Since the symbols = and == are defined as assignment and equality operators in Python, they cannot be used to formulate symbolic equations. SymPy provides Eq() function to set up an equation.
>>> from sympy import * >>> x,y=symbols('x y') >>> Eq(x,y)
The above code snippet gives an output equivalent to the below expression −
x = y
Since x=y is possible if and only if x-y=0, above equation can be written as −
>>> Eq(x-y,0)
The above code snippet gives an output equivalent to the below expression −
x − y = 0
The solver module in SymPy provides soveset() function whose prototype is as follows −
solveset(equation, variable, domain)
The domain is by default S.Complexes. Using solveset() function, we can solve an algebraic equation as follows −
>>> solveset(Eq(x**2-9,0), x)
The following output is obtained −
{−3, 3}
>>> solveset(Eq(x**2-3*x, -2),x)
The following output is obtained after executing the above code snippet −
{1,2}
The output of solveset is a FiniteSet of the solutions. If there are no solutions, an EmptySet is returned
>>> solveset(exp(x),x)
The following output is obtained after executing the above code snippet −
$\varnothing$
Linear equation
We have to use linsolve() function to solve linear equations.
For example, the equations are as follows −
x-y=4
x+y=1
>>> from sympy import * >>> x,y=symbols('x y') >>> linsolve([Eq(x-y,4),Eq( x + y ,1) ], (x, y))
The following output is obtained after executing the above code snippet −
$\lbrace(\frac{5}{2},-\frac{3}{2})\rbrace$
The linsolve() function can also solve linear equations expressed in matrix form.
>>> a,b=symbols('a b') >>> a=Matrix([[1,-1],[1,1]]) >>> b=Matrix([4,1]) >>> linsolve([a,b], (x,y))
We get the following output if we execute the above code snippet −
$\lbrace(\frac{5}{2},-\frac{3}{2})\rbrace$
Non-linear equation
For this purpose, we use nonlinsolve() function. Equations for this example −
a2+a=0 a-b=0
>>> a,b=symbols('a b') >>> nonlinsolve([a**2 + a, a - b], [a, b])
We get the following output if we execute the above code snippet −
$\lbrace(-1, -1),(0,0)\rbrace$
differential equation
First, create an undefined function by passing cls=Function to the symbols function. To solve differential equations, use dsolve.
>>> x=Symbol('x') >>> f=symbols('f', cls=Function) >>> f(x)
The following output is obtained after executing the above code snippet −
f(x)
Here f(x) is an unevaluated function. Its derivative is as follows −
>>> f(x).diff(x)
The above code snippet gives an output equivalent to the below expression −
$\frac{d}{dx}f(x)$
We first create Eq object corresponding to following differential equation
>>> eqn=Eq(f(x).diff(x)-f(x), sin(x)) >>> eqn
The above code snippet gives an output equivalent to the below expression −
$-f(x) + \frac{d}{dx}f(x)= \sin(x)$
>>> dsolve(eqn, f(x))
The above code snippet gives an output equivalent to the below expression −
$f(x)=(c^1-\frac{e^-xsin(x)}{2}-\frac{e^-xcos(x)}{2})e^x$