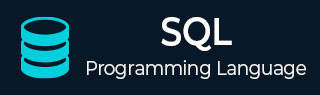
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Databases
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
SQL - Null Functions
SQL NULL functions are used to perform operations on NULL values that are stored in the database tables.
A NULL value serves as a placeholder in the database when data is absent or the required information is unavailable. It is a flexible value not associated to any specific data type and can be used in columns of various data types, including string, int, varchar, and more.
Following are the various features of a NULL value −
The NULL value is different from a zero value or a field containing a space. A record with a NULL value is one that has been left empty or unspecified during record creation.
The NULL value assists us in removing ambiguity from data. Thus, maintaining the uniform datatype across the column.
SQL NULL Functions
To handle these NULL values in a database table, SQL provides various NULL functions. They are listed as follows −
- ISNULL()
- COALESCE()
- NULLIF()
- IFNULL()
The ISNULL() Function
The SQL ISNULL() function returns 0 and 1 depending on whether the expression is null or not. If the expression is null, then this function returns 1; otherwise, it returns 0.
Syntax
Following is the syntax for the ISNULL() function −
ISNULL(column_name)
Example
First of all let us create a table named CUSTOMERS, containing the personal details of customers including their name, age, address and salary etc., using the following query −
CREATE TABLE CUSTOMERS ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, ADDRESS CHAR (25), SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
Now, insert records into this table using the INSERT INTO statement as follows −
INSERT INTO CUSTOMERS VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00 ), (2, 'Khilan', 25, 'Delhi', 1500.00 ), (3, 'Kaushik', 23, 'Kota', NULL ), (4, 'Chaitali', 25, 'Mumbai', 6500.00 ), (5, 'Hardik', 27, 'Bhopal', 8500.00 ), (6, 'Komal', 22, 'Hyderabad', NULL ), (7, 'Indore', 24, 'Indore', 10000.00 );
The table will be created as −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | NULL |
4 | Chaitali | 25 | Mumbai | 6500.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | NULL |
7 | Indore | 24 | Indore | 10000.00 |
Following is the query to check whether SALARY is NULL or not −
SELECT SALARY, ISNULL(SALARY) AS Null_value FROM CUSTOMERS;
Output
On execution of the above query, we get the column "SALARY" and Null_value. If the SALARY is NULL, then their null value is 1; otherwise, it is 0. −
SALARY | Null_value |
---|---|
2000.00 | 0 |
1500.00 | 0 |
NULL | 1 |
6500.00 | 0 |
8500.00 | 0 |
NULL | 1 |
10000.00 | 0 |
The COALESCE() Function
The SQL COALESCE() function returns the first occurred NON-NULL expression among its arguments. If all the expressions are NULL, then the COALESCE() function will return NULL.
An integer is evaluated first in the COALESCE() function, and an integer followed by a character expression always produces an integer as the output.
Syntax
Following is the syntax for the COALESCE() function −
COALESCE(expression_1, expression_2, expression_n);
Example
In the following query, we are returning the first occurred NON-NULL value −
SELECT COALESCE (NULL, 'welcome', 'tutorialspoint') AS Result;
Output
On executing the above query, we get "welcome" as a result, because it is the first NON-NULL value −
Result |
---|
welcome |
Example
In the following query, we are using the COALESCE() function on the SALARY and AGE columns of CUSTOMERS table. The first NON-NULL values evaluated from these two columns are displayed in another column named "Result".
SELECT NAME, SALARY, AGE, COALESCE(SALARY, AGE) AS Result FROM CUSTOMERS;
Output
When you execute the above query, we get the following table as a result −
NAME | SALARY | AGE | Result |
---|---|---|---|
Ramesh | 2000.00 | 32 | 2000.00 |
Khilan | 1500.00 | 25 | 1500.00 |
Kaushik | NULL | 23 | 23.00 |
Chaitali | 6500.00 | 25 | 6500.00 |
Hardik | 8500.00 | 27 | 8500.00 |
Komal | NULL | 22 | 22.00 |
Indore | 10000.00 | 24 | 10000.00 |
The NULLIF() Function
The SQL NULLIF() function compares two expressions. If both expressions are the same, it returns NULL. Otherwise, it returns the first expression. This function can be used directly with clauses like SELECT, WHERE, and GROUP BY.
Syntax
Following is the syntax of NULLIF() function −
NULLIF(expression_1, expression_2);
Example
The following SQL query uses NULLIF() function to compare values in NAME and ADDRESS columns of the CUSTOMERS table. If the NAME value matches the ADDRESS value, the result is NULL; otherwise, it returns the NAME value. The result values are stored in another column called "Result".
SELECT NAME, ADDRESS, NULLIF(NAME, ADDRESS) AS Result FROM CUSTOMERS;
Output
When you execute the above query, we get the following table as a result −
NAME | ADDRESS | Result |
---|---|---|
Ramesh | Ahmedabad | Ramesh |
Khilan | Delhi | Khilan |
Kaushik | Kota | Kaushik |
Chaitali | Mumbai | Chaitali |
Hardik | Bhopal | Hardik |
Komal | Hyderabad | Komal |
Indore | Indore | NULL |
The IFNULL() Function
The IFNULL() function replaces the NULL values in a database table with a specific value. This function accepts two arguments. If the first argument is a NULL value, it is replaced with the second argument. Otherwise, the first argument is returned as it is.
This function does not work in the SQL Server database.
If both the arguments are NULL, the result of this function is also NULL.
Syntax
Following is the syntax for IFNULL() function −
IFNULL(column_name, value_to_replace);
Example
The following query evaluates the values in SALARY column of the CUSTOMERS table. Using the IFNULL() function, we are replacing the NULL values in this column (if any) with the value 5500 −
SELECT NAME, SALARY, IFNULL(SALARY, 5500) AS Result FROM CUSTOMERS;
Output
Following is the output of the above query −
NAME | SALARY | Result |
---|---|---|
Ramesh | 2000.00 | 2000.00 |
Khilan | 1500.00 | 1500.00 |
Kaushik | NULL | 5500.00 |
Chaitali | 6500.00 | 6500.00 |
Hardik | 8500.00 | 8500.00 |
Komal | NULL | 5500.00 |
Indore | 10000.00 | 10000.00 |