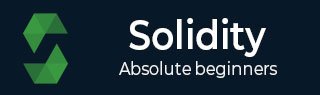
- Solidity Tutorial
- Solidity - Home
- Solidity - Overview
- Solidity - Environment Setup
- Solidity - Basic Syntax
- Solidity - First Application
- Solidity - Comments
- Solidity - Types
- Solidity - Variables
- Solidity - Variable Scope
- Solidity - Operators
- Solidity - Loops
- Solidity - Decision Making
- Solidity - Strings
- Solidity - Arrays
- Solidity - Enums
- Solidity - Structs
- Solidity - Mappings
- Solidity - Conversions
- Solidity - Ether Units
- Solidity - Special Variables
- Solidity - Style Guide
- Solidity Functions
- Solidity - Functions
- Solidity - Function Modifiers
- Solidity - View Functions
- Solidity - Pure Functions
- Solidity - Fallback Function
- Function Overloading
- Mathematical Functions
- Cryptographic Functions
- Solidity Common Patterns
- Solidity - Withdrawal Pattern
- Solidity - Restricted Access
- Solidity Advanced
- Solidity - Contracts
- Solidity - Inheritance
- Solidity - Constructors
- Solidity - Abstract Contracts
- Solidity - Interfaces
- Solidity - Libraries
- Solidity - Assembly
- Solidity - Events
- Solidity - Error Handling
- Solidity Useful Resources
- Solidity - Quick Guide
- Solidity - Useful Resources
- Solidity - Discussion
Solidity - if...else statement
The 'if...else' statement is the next form of control statement that allows Solidity to execute statements in a more controlled way.
Syntax
if (expression) { Statement(s) to be executed if expression is true } else { Statement(s) to be executed if expression is false }
Here Solidity expression is evaluated. If the resulting value is true, the given statement(s) in the 'if' block, are executed. If the expression is false, then the given statement(s) in the else block are executed.
Example
pragma solidity ^0.5.0; contract SolidityTest { uint storedData; constructor() public{ storedData = 10; } function getResult() public view returns(string memory){ uint a = 1; uint b = 2; uint result if( a > b) { // if else statement result = a; } else { result = b; } return integerToString(result); } function integerToString(uint _i) internal pure returns (string memory) { if (_i == 0) { return "0"; } uint j = _i; uint len; while (j != 0) { len++; j /= 10; } bytes memory bstr = new bytes(len); uint k = len - 1; while (_i != 0) { bstr[k--] = byte(uint8(48 + _i % 10)); _i /= 10; } return string(bstr);//access local variable } }
Run the above program using steps provided in Solidity First Application chapter.
Output
0: string: 2
solidity_decision_making.htm
Advertisements