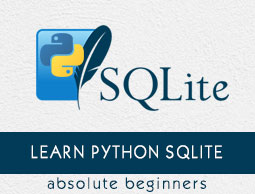
- Python SQLite Tutorial
- Python SQLite - Home
- Python SQLite - Introduction
- Python SQLite - Establishing Connection
- Python SQLite - Create Table
- Python SQLite - Insert Data
- Python SQLite - Select Data
- Python SQLite - Where Clause
- Python SQLite - Order By
- Python SQLite - Update Table
- Python SQLite - Delete Data
- Python SQLite - Drop Table
- Python SQLite - Limit
- Python SQLite - Join
- Python SQLite - Cursor Object
- Python SQLite Useful Resources
- Python SQLite - Quick Guide
- Python SQLite - Useful Resources
- Python SQLite - Discussion
Python SQLite - Cursor Object
The sqlite3.Cursor class is an instance using which you can invoke methods that execute SQLite statements, fetch data from the result sets of the queries. You can create Cursor object using the cursor() method of the Connection object/class.
Example
import sqlite3 #Connecting to sqlite conn = sqlite3.connect('example.db') #Creating a cursor object using the cursor() method cursor = conn.cursor()
Methods
Following are the various methods provided by the Cursor class/object.
Method | Description |
---|---|
execute() |
This routine executes an SQL statement. The SQL statement may be parameterized (i.e., placeholders instead of SQL literals). The psycopg2 module supports placeholder using %s sign For example:cursor.execute("insert into people values (%s, %s)", (who, age)) |
executemany() |
This routine executes an SQL command against all parameter sequences or mappings found in the sequence sql. |
fetchone() |
This method fetches the next row of a query result set, returning a single sequence, or None when no more data is available. |
fetchmany() |
This routine fetches the next set of rows of a query result, returning a list. An empty list is returned when no more rows are available. The method tries to fetch as many rows as indicated by the size parameter. |
fetchall() |
This routine fetches all (remaining) rows of a query result, returning a list. An empty list is returned when no rows are available. |
Properties
Following are the properties of the Cursor class −
Method | Description |
---|---|
arraySize |
This is a read/write property you can set the number of rows returned by the fetchmany() method. |
description |
This is a read only property which returns the list containing the description of columns in a result-set. |
lastrowid |
This is a read only property, if there are any auto-incremented columns in the table, this returns the value generated for that column in the last INSERT or, UPDATE operation. |
rowcount |
This returns the number of rows returned/updated in case of SELECT and UPDATE operations. |
connection |
This read-only attribute provides the SQLite database Connection used by the Cursor object. |