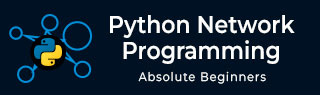
- Python - Network Programming
- Python - Network Introduction
- Python - Network Environment
- Python - Internet Protocol
- Python - IP Address
- Python - DNS Lookup
- Python - Routing
- Python - HTTP Requests
- Python - HTTP Response
- Python - HTTP Headers
- Python - Custom HTTP Requests
- Python - Request Status Codes
- Python - HTTP Authentication
- Python - HTTP Data Download
- Python - Connection Re-use
- Python - Network Interface
- Python - Sockets Programming
- Python - HTTP Client
- Python - HTTP Server
- Python - Building URLs
- Python - WebForm Submission
- Python - Databases and SQL
- Python - Telnet
- Python - Email Messages
- Python - SMTP
- Python - POP3
- Python - IMAP
- Python - SSH
- Python - FTP
- Python - SFTP
- Python - Web Servers
- Python - Uploading Data
- Python - Proxy Server
- Python - Directory Listing
- Python - Remote Procedure Call
- Python - RPC JSON Server
- Python - Google Maps
- Python - RSS Feed
Python - Telnet
Telnet is a type of network protocol which allows a user in one computer to logon to another computer which also belongs to the same network. The telnet command is used along with the host name and then the user credentials are entered. Upon successful login the remote user can access the applications and data in a way similar to the regular user of the system. Of course some privileges can be controlled by the administrator of the system who sets up and maintains the system.
In Python telnet is implemented by the module telnetlib which has the Telnet class which has the required methods to establish the connection. In the below example we also use the getpass module to handle the password prompt as part of the login process. Also we assume the connection is made to a unix host. The various methods from telnetlib.Telnet class used in the program are explained below.
Telnet.read_until - Read until a given string, expected, is encountered or until timeout seconds have passed.
Telnet.write - Write a string to the socket, doubling any IAC characters. This can block if the connection is blocked. May raise socket.error if the connection is closed.
Telnet.read_all() - Read all data until EOF; block until connection closed.
Example
import getpass import telnetlib HOST = "http://localhost:8000/" user = raw_input("Enter your remote account: ") password = getpass.getpass() tn = telnetlib.Telnet(HOST) tn.read_until("login: ") tn.write(user + "\n") if password: tn.read_until("Password: ") tn.write(password + "\n") tn.write("ls\n") tn.write("exit\n") print tn.read_all()
When we run the above program, we get the following output −
- lrwxrwxrwx 1 0 0 1 Nov 13 2012 ftp -> . - lrwxrwxrwx 1 0 0 3 Nov 13 2012 mirror -> pub - drwxr-xr-x 23 0 0 4096 Nov 27 2017 pub - drwxr-sr-x 88 0 450 4096 May 04 19:30 site - drwxr-xr-x 9 0 0 4096 Jan 23 2014 vol
Please note that this output is specific to the remote computer whose details are submitted when the program is run.