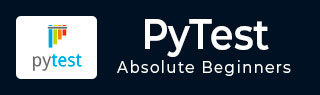
- Pytest Tutorial
- Pytest - Home
- Pytest - Introduction
- Pytest - Environment Setup
- Identifying Test files and Functions
- Pytest - Starting With Basic Test
- Pytest - File Execution
- Execute a Subset of Test Suite
- Substring Matching of Test Names
- Pytest - Grouping the Tests
- Pytest - Fixtures
- Pytest - Conftest.py
- Pytest - Parameterizing Tests
- Pytest - Xfail/Skip Tests
- Stop Test Suite after N Test Failures
- Pytest - Run Tests in Parallel
- Test Execution Results in XML
- Pytest - Summary
- Pytest - Conclusion
- Pytest useful Resources
- Pytest - Quick Guide
- Pytest - Useful Resources
- Pytest - Discussion
Pytest - Grouping the Tests
In this chapter, we will learn how to group the tests using markers.
Pytest allows us to use markers on test functions. Markers are used to set various features/attributes to test functions. Pytest provides many inbuilt markers such as xfail, skip and parametrize. Apart from that, users can create their own marker names. Markers are applied on the tests using the syntax given below −
@pytest.mark.<markername>
To use markers, we have to import pytest module in the test file. We can define our own marker names to the tests and run the tests having those marker names.
To run the marked tests, we can use the following syntax −
pytest -m <markername> -v
-m <markername> represents the marker name of the tests to be executed.
Update our test files test_compare.py and test_square.py with the following code. We are defining 3 markers – great, square, others.
test_compare.py
import pytest @pytest.mark.great def test_greater(): num = 100 assert num > 100 @pytest.mark.great def test_greater_equal(): num = 100 assert num >= 100 @pytest.mark.others def test_less(): num = 100 assert num < 200
test_square.py
import pytest import math @pytest.mark.square def test_sqrt(): num = 25 assert math.sqrt(num) == 5 @pytest.mark.square def testsquare(): num = 7 assert 7*7 == 40 @pytest.mark.others def test_equality(): assert 10 == 11
Now to run the tests marked as others, run the following command −
pytest -m others -v
See the result below. It ran the 2 tests marked as others.
test_compare.py::test_less PASSED test_square.py::test_equality FAILED ============================================== FAILURES ============================================== ___________________________________________ test_equality ____________________________________________ @pytest.mark.others def test_equality(): > assert 10 == 11 E assert 10 == 11 test_square.py:16: AssertionError ========================== 1 failed, 1 passed, 4 deselected in 0.08 seconds ==========================
Similarly, we can run tests with other markers also – great, compare