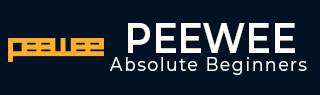
- Peewee Tutorial
- Peewee - Home
- Peewee - Overview
- Peewee - Database Class
- Peewee - Model
- Peewee - Field Class
- Peewee - Insert a New Record
- Peewee - Select Records
- Peewee - Filters
- Peewee - Primary & Composite Keys
- Peewee - Update Existing Records
- Peewee - Delete Records
- Peewee - Create Index
- Peewee - Constraints
- Peewee - Using MySQL
- Peewee - Using PostgreSQL
- Peewee - Defining Database Dynamically
- Peewee - Connection Management
- Peewee - Relationships & Joins
- Peewee - Subqueries
- Peewee - Sorting
- Peewee - Counting & Aggregation
- Peewee - SQL Functions
- Peewee - Retrieving Row Tuples/Dictionaries
- Peewee - User defined Operators
- Peewee - Atomic Transactions
- Peewee - Database Errors
- Peewee - Query Builder
- Peewee - Integration with Web Frameworks
- Peewee - SQLite Extensions
- Peewee - PostgreSQL & MySQL Extensions
- Peewee - Using CockroachDB
- Peewee Useful Resources
- Peewee - Quick Guide
- Peewee - Useful Resources
- Peewee - Discussion
Peewee - User defined Operators
Peewee has Expression class with the help of which we can add any customized operator in Peewee’s list of operators. Constructor for Expression requires three arguments, left operand, operator and right operand.
op=Expression(left, operator, right)
Using Expression class, we define a mod() function that accepts arguments for left and right and ‘%’ as operator.
from peewee import Expression # the building block for expressions def mod(lhs, rhs): return Expression(lhs, '%', rhs)
Example
We can use it in a SELECT query to obtain list of records in Contacts table with even id.
from peewee import * db = SqliteDatabase('mydatabase.db') class BaseModel(Model): class Meta: database = db class Contacts(BaseModel): RollNo = IntegerField() Name = TextField() City = TextField() db.create_tables([Contacts]) from peewee import Expression # the building block for expressions def mod(lhs, rhs): return Expression(lhs,'%', rhs) qry=Contacts.select().where (mod(Contacts.id,2)==0) print (qry.sql()) for q in qry: print (q.id, q.Name, q.City)
This code will emit following SQL query represented by the string −
('SELECT "t1"."id", "t1"."RollNo", "t1"."Name", "t1"."City" FROM "contacts" AS "t1" WHERE (("t1"."id" % ?) = ?)', [2, 0])
Output
Therefore, the output is as follows −
2 Amar Delhi 4 Leena Nasik 6 Hema Nagpur 8 John Delhi 10 Raja Nasik
Advertisements