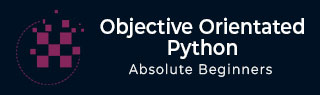
- Object Oriented Python Tutorial
- Home
- Introduction
- Environment Setup
- Data Structures
- Building Blocks
- Object Oriented Shortcuts
- Inheritance and Ploymorphism
- Python Design Pattern
- Advanced Features
- Files and Strings
- Exception and Exception Classes
- Object Serialization
- Python Libraries
- Object Oriented Python Resources
- Quick Guide
- Useful Resources
- Discussion
Exception and Exception Classes
In general, an exception is any unusual condition. Exception usually indicates errors but sometimes they intentionally puts in the program, in cases like terminating a procedure early or recovering from a resource shortage. There are number of built-in exceptions, which indicate conditions like reading past the end of a file, or dividing by zero. We can define our own exceptions called custom exception.
Exception handling enables you handle errors gracefully and do something meaningful about it. Exception handling has two components: “throwing” and ‘catching’.
Identifying Exception (Errors)
Every error occurs in Python result an exception which will an error condition identified by its error type.
>>> #Exception >>> 1/0 Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> 1/0 ZeroDivisionError: division by zero >>> >>> var = 20 >>> print(ver) Traceback (most recent call last): File "<pyshell#5>", line 1, in <module> print(ver) NameError: name 'ver' is not defined >>> #Above as we have misspelled a variable name so we get an NameError. >>> >>> print('hello) SyntaxError: EOL while scanning string literal >>> #Above we have not closed the quote in a string, so we get SyntaxError. >>> >>> #Below we are asking for a key, that doen't exists. >>> mydict = {} >>> mydict['x'] Traceback (most recent call last): File "<pyshell#15>", line 1, in <module> mydict['x'] KeyError: 'x' >>> #Above keyError >>> >>> #Below asking for a index that didn't exist in a list. >>> mylist = [1,2,3,4] >>> mylist[5] Traceback (most recent call last): File "<pyshell#20>", line 1, in <module> mylist[5] IndexError: list index out of range >>> #Above, index out of range, raised IndexError.
Catching/Trapping Exception
When something unusual occurs in your program and you wish to handle it using the exception mechanism, you ‘throw an exception’. The keywords try and except are used to catch exceptions. Whenever an error occurs within a try block, Python looks for a matching except block to handle it. If there is one, execution jumps there.
syntax
try: #write some code #that might throw some exception except <ExceptionType>: # Exception handler, alert the user
The code within the try clause will be executed statement by statement.
If an exception occurs, the rest of the try block will be skipped and the except clause will be executed.
try: some statement here except: exception handling
Let’s write some code to see what happens when you not use any error handling mechanism in your program.
number = int(input('Please enter the number between 1 & 10: ')) print('You have entered number',number)
Above programme will work correctly as long as the user enters a number, but what happens if the users try to puts some other data type(like a string or a list).
Please enter the number between 1 > 10: 'Hi' Traceback (most recent call last): File "C:/Python/Python361/exception2.py", line 1, in <module> number = int(input('Please enter the number between 1 & 10: ')) ValueError: invalid literal for int() with base 10: "'Hi'"
Now ValueError is an exception type. Let’s try to rewrite the above code with exception handling.
import sys print('Previous code with exception handling') try: number = int(input('Enter number between 1 > 10: ')) except(ValueError): print('Error..numbers only') sys.exit() print('You have entered number: ',number)
If we run the program, and enter a string (instead of a number), we can see that we get a different result.
Previous code with exception handling Enter number between 1 > 10: 'Hi' Error..numbers only
Raising Exceptions
To raise your exceptions from your own methods you need to use raise keyword like this
raise ExceptionClass(‘Some Text Here’)
Let’s take an example
def enterAge(age): if age<0: raise ValueError('Only positive integers are allowed') if age % 2 ==0: print('Entered Age is even') else: print('Entered Age is odd') try: num = int(input('Enter your age: ')) enterAge(num) except ValueError: print('Only positive integers are allowed')
Run the program and enter positive integer.
Expected Output
Enter your age: 12 Entered Age is even
But when we try to enter a negative number we get,
Expected Output
Enter your age: -2 Only positive integers are allowed
Creating Custom exception class
You can create a custom exception class by Extending BaseException class or subclass of BaseException.
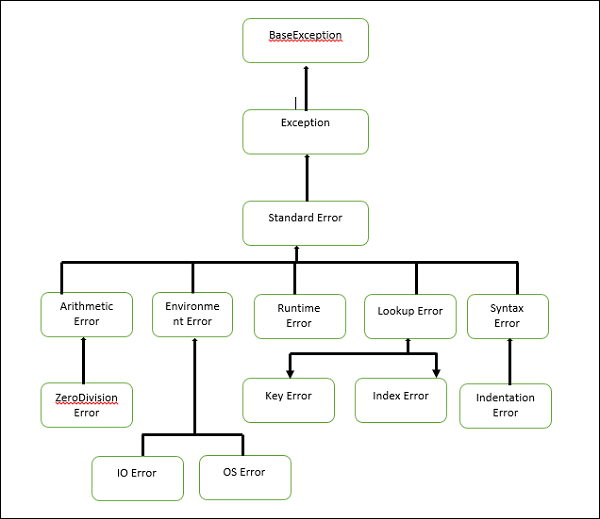
From above diagram we can see most of the exception classes in Python extends from the BaseException class. You can derive your own exception class from BaseException class or from its subclass.
Create a new file called NegativeNumberException.py and write the following code.
class NegativeNumberException(RuntimeError): def __init__(self, age): super().__init__() self.age = age
Above code creates a new exception class named NegativeNumberException, which consists of only constructor which call parent class constructor using super()__init__() and sets the age.
Now to create your own custom exception class, will write some code and import the new exception class.
from NegativeNumberException import NegativeNumberException def enterage(age): if age < 0: raise NegativeNumberException('Only positive integers are allowed') if age % 2 == 0: print('Age is Even') else: print('Age is Odd') try: num = int(input('Enter your age: ')) enterage(num) except NegativeNumberException: print('Only positive integers are allowed') except: print('Something is wrong')
Output
Enter your age: -2 Only positive integers are allowed
Another way to create a custom Exception class.
class customException(Exception): def __init__(self, value): self.parameter = value def __str__(self): return repr(self.parameter) try: raise customException('My Useful Error Message!') except customException as instance: print('Caught: ' + instance.parameter)
Output
Caught: My Useful Error Message!
Exception hierarchy
The class hierarchy for built-in exceptions is −
+-- SystemExit +-- KeyboardInterrupt +-- GeneratorExit +-- Exception +-- StopIteration +-- StopAsyncIteration +-- ArithmeticError | +-- FloatingPointError | +-- OverflowError | +-- ZeroDivisionError +-- AssertionError +-- AttributeError +-- BufferError +-- EOFError +-- ImportError +-- LookupError | +-- IndexError | +-- KeyError +-- MemoryError +-- NameError | +-- UnboundLocalError +-- OSError | +-- BlockingIOError | +-- ChildProcessError | +-- ConnectionError | | +-- BrokenPipeError | | +-- ConnectionAbortedError | | +-- ConnectionRefusedError | | +-- ConnectionResetError | +-- FileExistsError | +-- FileNotFoundError | +-- InterruptedError | +-- IsADirectoryError | +-- NotADirectoryError | +-- PermissionError | +-- ProcessLookupError | +-- TimeoutError +-- ReferenceError +-- RuntimeError | +-- NotImplementedError | +-- RecursionError +-- SyntaxError | +-- IndentationError | +-- TabError +-- SystemError +-- TypeError +-- ValueError | +-- UnicodeError | +-- UnicodeDecodeError | +-- UnicodeEncodeError | +-- UnicodeTranslateError +-- Warning +-- DeprecationWarning +-- PendingDeprecationWarning +-- RuntimeWarning +-- SyntaxWarning +-- UserWarning +-- FutureWarning +-- ImportWarning +-- UnicodeWarning +-- BytesWarning +-- ResourceWarning