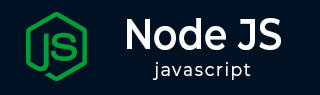
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS v8.serializer.writeRawBytes() Method
The NodeJS v8.serializer.writeRawBytes() method of class v8.serializer is used to write raw bytes into the serializer's internal buffer. The term “raw byte" means an uninterpreted byte values.
Syntax
Following is the syntax of the NodeJS v8.serializer.writeRawBytes() method −
v8.serializer.writeRawBytes(buffer)
Parameters
This method accepts only one parameter.
buffer − A Buffer, TypedArray, DataView that will be written to the internal buffer.
Return Value
This method returns undefined instead, it Writes raw bytes into the serializer's internal buffer.
Example
In the example, we are trying to write raw bytes into the serializer's internal buffer using NodeJS v8.serializer.writeRawBytes() method.
const v8 = require('v8'); const serializer = new v8.Serializer(); // Writing raw bytes after serializing with v8 console.log(serializer.writeRawBytes(v8.serialize('TutorialsPoint')));
Output
As we can see in the output below, the writeRawBytes() method returns undefined instead, it writes the raw bytes (that are passed as parameter) to the internal buffer.
undefined
Example
In the following example below, we are trying to write raw bytes to the serializer's internal buffer. Then we are releasing the internal buffer to check whether the raw bytes are written or not.
const v8 = require('v8'); const serializer = new v8.Serializer(); let data = 'TutorialsPoint'; // Writing raw bytes after serializing console.log(serializer.writeRawBytes(v8.serialize(data))); console.log(serializer.releaseBuffer());
Output
undefined <Buffer ff 0d 22 0e 54 75 74 6f 72 69 61 6c 73 50 6f 69 6e 74>
Example
In this example, we are trying to pass a typedArray as a rawbytes that will be written to the serializer's internal buffer.
const v8 = require('v8'); const serializer = new v8.Serializer(); const typedArray = new Int8Array(4); typedArray[0] = 5; typedArray[1] = 7; typedArray[2] = 9; typedArray[3] = 11; console.log(serializer.writeRawBytes(v8.serialize(typedArray))); console.log(serializer.releaseBuffer());
Output
undefined <Buffer ff 0d 5c 00 04 05 07 09 0b>
To Continue Learning Please Login