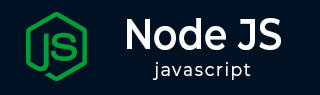
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - url.toString() Method
The NodeJS url.toString() method of URL class will return the serialized URL from the URL object. The returned value of this method is equivalent to the URL.href property and URL.toJSON() method. If the value assigned to toString() method is not a valid URL, a TypeError will be thrown.
There are several utilities for URL resolution and parsing provided by the Node.js URL module and toString() method is one of it.
Syntax
Following is the syntax of the NodeJS toString() method of URL class
URL.toString()
Parameters
This method does not accept any parameters.
Return Value
This method on the URL object returns the serialized URL.
Example
If we try to assign a valid URL to the NodeJS url.toString() method, it returns a serialized URL as a string.
In the example below, we are trying to get the serialized URL from the URL object.
const url = require('node:url'); const myURL = new URL("https://www.tutorialspoint.com/index.htm"); var result = myURL.toString(); console.log("The serialized URL: " + result);
Output
After executing the above program, the url.toString() method returns serialized URL string.
The serialized URL: https://www.tutorialspoint.com/index.htm
Example
If we assign a valid URL to the href property and toString() method, the returned values of both are equivalent.
In the following example, we are assigning a valid URL to the href property and toString method and checking if both are equivalent.
const url = require('node:url'); const myURL = new URL("https://www.tutorialspoint.com/index.htm"); var toString = myURL.toString(); console.log("Result of toString property: " + toString); var href = myURL.href; console.log("Result of href property: " + href); if(toString === href){ console.log("Both toString and href results are same..."); } else { console.log("Both are not same..."); }
Output
As we can see in the output below, the returned values are same.
Result of toString property: https://www.tutorialspoint.com/index.htm Result of href property: https://www.tutorialspoint.com/index.htm Both toString and href results are same...
Example
If we assign a value that is not a valid URL type, then toString() method will throw a TypeError.
In the given program, we are assigning an invalid URL to the toString method.
const url = require('node:url'); const myURL = new URL("234httttttqerps://www.tutorialspoint.cgfom/index.htm"); var result = myURL.toString(); console.log("The serialized URL: " + result);
TypeError
If we compile and run the above program, the toString() method throws a TypeError as the assigned URL is not valid.
node:internal/url:564 throw new ERR_INVALID_URL(input); ^ TypeError [ERR_INVALID_URL]: Invalid URL at new NodeError (node:internal/errors:387:5) at URL.onParseError (node:internal/url:564:9) at new URL (node:internal/url:640:5) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:15) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 { input: '234httttttqerps://www.tutorialspoint.cgfom/index.htm', code: 'ERR_INVALID_URL' }