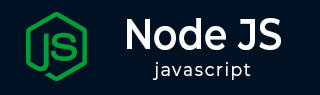
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - url.toJSON() Method
The NodeJS url.toJSON() method of URL class will return the serialized URL from the URL object. The returned value of this method is equivalent to the URL.href property and URL.toString() method. A TypeError will be thrown, if the value assigned to toJSON() method is not a valid URL.
The URL module of Node.js provides various utilities for URL resolution and parsing and toJSON() method is one in that.
Syntax
Following is the syntax of the NodeJS toJSON() method of URL class
URL.toJSON()
Parameters
This method does not accept any parameters.
Return Value
This method returns the serialized URL of the URL object.
Example
The NodeJS url.toJSON() method returns a serialized URL as a string, if we try to assign it a valid URL.
In the example below, we are trying to get the serialized URL from the URL object.
const url = require('node:url'); const myURL = new URL("https://www.tutorialspoint.com/index.htm"); console.log("The serialized URL: " + myURL.toJSON());
Output
As we can see in the output below, the NodeJS url.toJSON() method returns a serialized URL string.
The serialized URL: https://www.tutorialspoint.com/index.htm
Example
If we assign a valid URL to the href property and toJSON() method, the returned values of both are equivalent.
In the following example, we are assigning a valid URL to the href property and toJSON method and checking if both are equivalent.
const url = require('node:url'); const myURL = new URL("https://www.tutorialspoint.com/index.htm"); var toJSON = myURL.toJSON(); console.log("Result of toJSON property: " + toJSON); var href = myURL.href; console.log("Result of href property: " + href); if(toJSON === href){ console.log("Both toJSON and href results are same..."); } else { console.log("Both are not same..."); }
Output
As we can see in the output below, the returned values are same.
Result of toJSON property: https://www.tutorialspoint.com/index.htm Result of href property: https://www.tutorialspoint.com/index.htm Both toJSON and href results are same...
Example
If we assign a value that is not a valid URL type, then toJSON() method will throw a TypeError.
In the following example, we are assigning an invalid URL to the toJSON() method.
const url = require('node:url'); const myURL = new URL("696wsfsps://www.tutor4523ialspoint.cgfom/ind1234ex.htm"); var result = myURL.toJSON(); console.log("The serialized URL: " + result);
TypeError
If we compile and run the above program, the toJSON() method throws a TypeError as the assigned URL is not valid.
node:internal/url:564 throw new ERR_INVALID_URL(input); ^ TypeError [ERR_INVALID_URL]: Invalid URL at new NodeError (node:internal/errors:387:5) at URL.onParseError (node:internal/url:564:9) at new URL (node:internal/url:640:5) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:15) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 { input: '696wsfsps://www.tutor4523ialspoint.cgfom/ind1234ex.htm', code: 'ERR_INVALID_URL' }
To Continue Learning Please Login