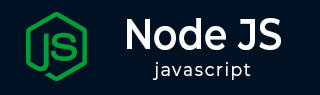
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - urlSearchParams.sort() Method
The NodeJS urlSearchParams.sort() method of class URLSearchParams is used to sort all the names of the name-value pairs in the query string and the sorting is done with a stable sorting algorithm (i.e. the relative order between name-value pairs with the same name will be preserved). This method sorts in ascending order.
This method returns undefined, to retrieve the result of this method assign the sorted query string to the toString() method.
The URLSearchParams API provides methods that give access to read and write the query of a URL. This class is also available on the global object.
Syntax
Following is the syntax of the NodeJS URLSearchParams.sort() method
URLSearchParams.sort()
Parameters
This method does not accept any parameters.
Return Value
This method returns undefined.
Following are the examples where we demonstrated the usage of the sort() method in different scenarios:
Example
If we assign a query string that contains name-value pairs to the NodeJS urlSearchParams.sort() method, it will sort all the name-value pairs by their names.
In the following example, we are trying to sort the name-value pairs in the input query string.
const url = require('node:url'); const myUrl = new URL('https://www.tutorialspoint.com?Monday=1&Thursday=4&Friday=5'); console.log("URL: " + myUrl.href); const Params = new URLSearchParams('Monday=1&Thursday=4&Friday=5'); console.log("Query string: " + Params); console.log(Params.sort()); console.log("The query string after sorting: " + Params.toString());
Output
As we can see in the output below, the name-value pairs were all sorted by their names.
URL: https://www.tutorialspoint.com/?Monday=1&Thursday=4&Friday=5 Query string: Monday=1&Thursday=4&Friday=5 undefined The query string after sorting: Friday=5&Monday=1&Thursday=4
Example
If there are multiple occurrences of a name in name-value pairs, the NodeJS sort() method will consider the first appeared name to be placed first according to the order.
In the program below, we are trying to sort the name-value pairs in the query string.
const url = require('node:url'); const myUrl = new URL('https://www.tutorialspoint.com?1=twoe&6=six&1=one&5=five'); console.log("URL: " + myUrl.href); const Params = new URLSearchParams('1=two&6=six&1=one&5=five'); console.log("Query string: " + Params); console.log(Params.sort()); console.log("The query string after sorting: " + Params.toString());
Output
As we can see in the output below, there are multiple appearances of the name ‘1 in the query string, but the sort() method considered the first occurrence of the name ‘1’ to place first according to the order.
URL: https://www.tutorialspoint.com/?1=twoe&6=six&1=one&5=five Query string: 1=two&6=six&1=one&5=five undefined The query string after sorting: 1=two&1=one&5=five&6=six
Example
The sort() method will sort the integers first followed by the special characters and then followed by the alphabet according to the ASCII sort order.
In the following example, the names of the name-value pairs in the input string are started with integers and a few of them started with special characters. We are trying to sort the name-value pairs by their names.
const url = require('node:url'); const myUrl = new URL('https://www.tutorialspoint.com?1=two&6=six&1=one&5=five'); console.log("URL: " + myUrl.href); const Params = new URLSearchParams('`1=two&6=six&1=one&5=five'); console.log("Query string: " + Params); console.log(Params.sort()); console.log("The query string after sorting: " + Params.toString());
Output
As we can see in the output, the names started with integers are sorted first followed by the special characters.
URL: https://www.tutorialspoint.com/?1=two&6=six&1=one&5=five Query string: %601=two&6=six&1=one&5=five Undefined The query string after sorting: 1=one&5=five&6=six&%601=two
To Continue Learning Please Login