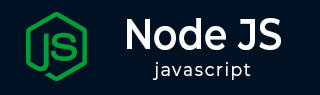
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - urlSearchParams.keys() Method
The NodeJS urlSearchParams.keys() method of class URLSearchParams returns an ES6 iterator that allows iteration through all the names of each name-value pair.
The URLSearchParams API provides methods that gives access to read and write the query of a URL. This class is also available on the global object.
Let’s consider a YouTube URL (‘https://www.youtube.com/watch?t=RS?f=TS&g=FR), where the portion after the ‘?’ is known as the query segment. In this query, (t) is the name, and (RS) is the value. Together it forms a name-value pair. There are three name-value pairs in the query string. So if we assign the query string to the key() method, it returns an ES6 iterator over the names of each name-value pair.
Syntax
Following is the syntax of the NodeJS URLSearchParams.keys() method
URLSearchParams.keys()
Parameters
This method does not accept any parameters.
Return Value
This method returns an ES6 iterator over the names of each name-value pair.
The following examples demonstrate the usage of the NodeJS URLSearchParams.keys() method:
Example
If the input URL string contains a query segment, the NodeJS urlSearchParams.keys() method returns an iterator over the names of name-value pairs from the query string.
In the following example, we are trying to get the names from the name-value pairs of the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?Monday=1&Thursday=4&Friday=5'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('monday=1&thursday=4&friday=5'); console.log("Query string: " + Params); console.log('All the names in the query string are: '); for (const name of Params.keys()) { console.log(name); }
Output
As we can see in the below output, the NodeJS keys() method returns all the names from the name-value pairs.
URL: https://www.tutorialspoint.com/?Monday=1&Thursday=4&Friday=5 Query string: monday=1&thursday=4&friday=5 All the names in the query string are: monday thursday friday
Example
In the following example, we are appending some name-value pairs to the input query string. Then we are trying to get the names from the name-value pairs.
const url = require('node:url'); const Params = new URLSearchParams('Monday=1&Thursday=4&Friday=5'); console.log("Query string: " + Params); Params.append('Saturday', 6); Params.append('Sunday', 7); console.log('All the names in the query string are: '); for (const name of Params.keys()) { console.log(name); }
Output
On executing the above program, it will generate the following output
Query string: Monday=1&Thursday=4&Friday=5 All the names in the query string are: Monday Thursday Friday Saturday Sunday