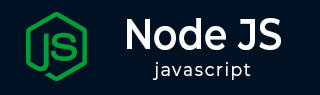
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - urlSearchParams.has() Method
The NodeJS urlSearchParams.has() method of URLSearchParams class prints true if the name passed to this method is present, else it prints false.
The URLSearchParams API provides methods that gives access to read and write the query of a URL. This class is also available on the global object.
Let’s look into an example to understand the has() method in a better way. Consider a ‘Search Engine’ in NETFLIX and we are trying to search for a particular movie in it. If it is present, it will show you that particular movie, else it displays an error message.
Syntax
Following is the syntax of the NodeJS URLSearchParams.has() method
URLSearchParams.has(name)
Parameters
name: This specifies the name of the parameter to find.
Return Value
This method returns a Boolean value.
The following examples demonstrate the usage of the NodeJS URLSearchParams.has() method:
Example
If the name passed to the NodeJS urlSearchParams.has() method is present in the query string, it returns true.
In the following example, we are trying to check if the name ‘header’ is present in the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?title=1&header=2&body=3&footer=4'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('title=1&header=2&body=3&footer=4'); console.log("Query string: " + Params); console.log("The name 'header' is present in query: " + Params.has("header"));
Output
As we can see in the output, the NodeJS has() method returned true because the name ‘header’ is in the query string.
URL: https://www.tutorialspoint.com/?title=1&header=2&body=3&footer=4 Query string: title=1&header=2&body=3&footer=4 The name 'header' is present in query: true
Example
If the name passed to the has() method is not present in the query string, it returns false.
In the following example below, we are trying to find whether the name "header" is in the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?title=1&header=2&body=3&footer=4'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('title=1&header=2&body=3&footer=4'); console.log("Query string: " + Params); console.log("The name 'contactUS' is present in query: " + Params.has("ContactUS"));
Output
After executing the above program, the has() method returns false because the name which has been searched is not present in the query string.
URL: https://www.tutorialspoint.com/?title=1&header=2&body=3&footer=4 Query string: title=1&header=2&body=3&footer=4 The name 'contactUS' is present in query: false