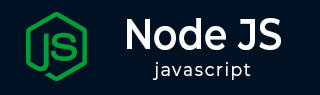
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - urlSearchParams.get() Method
The NodeJS urlSearchParams.get() method of URLSearchParams class retrieves the value from a specified name in the query string.
The query portion of the URL can be read and written using the URLSearchParams API. This class is also available on the global object.
To get a better understanding of this method, let’s look into a real-life example. Consider this URL (‘https://www.youtube.com/watch?v=towpH780PT0’), where 'v=towpH780PT0' is known as the query segment. In this query, (v) is the name, and (towpH780PT0) is the value. Together it forms a name-value pair.
Syntax
Following is the syntax of the NodeJS URLSearchParams.get() method
URLSearchParams.get(name)
Parameters
name: This specifies the name of the parameter to return.
Return Value
This method returns a string value of the given name passed as a parameter.
If there are multiple name-value pairs with the given name, this method returns the value in the first pair.
If there is no pair whose name is name, null is returned.
Example
In the following example, we are trying to get the value of the key named ‘two’ in the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?one=1&two=2&three=3'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('one=1&two=2&three=3'); console.log("Query string: " + Params); console.log("Trying to get the value of key 'two'....."); console.log("The value is: " + Params.get("two"));
Output
After executing the above program, the NodeJS get() method returns the value of the key ‘two’.
URL: https://www.tutorialspoint.com/?one=1&two=2&three=3 Query string: one=1&two=2&three=3 Trying to get the value of key 'two'..... The value is: 2
Example
If the name to be searched is present multiple times in the query string, the get() method returns the value of its first occurrence.
In the program below, we are trying to get the value of the name ‘two’ in the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?two=1&two=2&two=3'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('two=1&two=2&two=3'); console.log("Query string: " + Params); console.log("Trying to get the value of the first key 'two'....."); console.log("The value is: " + Params.get("two"));
Output
As we can see in the output, the get() method returns the value of the first occurrence.
URL: https://www.tutorialspoint.com/?two=1&two=2&two=3 Query string: two=1&two=2&two=3 Trying to get the value of the first key 'two'..... The value is: 1
Example
If the name to be searched is not present in the query string, the get() method returns null.
In the example below, we are trying to get the value of a name that is not present in the query string.
const url = require('node:url'); const MyUrl = new URL('https://www.tutorialspoint.com?HTML=499&NODEJS=999&CSS=699'); console.log("URL: ", MyUrl.href); const Params = new URLSearchParams('HTML=499&NODEJS=999&CSS=699'); console.log("Query string: " + Params); console.log("Trying to get the value of the first key 'JavaScript'....."); console.log("The value is: " + Params.get("JavaScript"));
Output
As we can see in the output, the get() method returns null.
URL: https://www.tutorialspoint.com/?HTML=499&NODEJS=999&CSS=699 Query string: HTML=499&NODEJS=999&CSS=699 Trying to get the value of the first key 'JavaScript'..... The value is: null