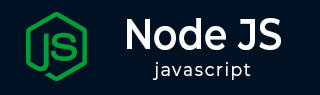
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - URLSearchParams.delete() Method
The NodeJS urlSearchParams.delete() method of class URLSearchParams accepts a name as a parameter and removes all its name/value pairs whose name is the name from the query string.
The query portion of the URL can be read and written using the URLSearchParams API. This class is also available on the global object.
Syntax
Following is the syntax of the NodeJS URLSearchParams.delete() method
URLSearchParams.delete(name)
Parameters
name: This parameter specifies the name to be deleted.
Return Value
This method removes all the name/value pairs whose name is name.
Example
If we pass a name to the NodeJS urlSearchParams.delete() method, it will remove that name/value pair from the input query string.
In the following example, we are trying to delete a name/value pair from the query string by passing the name to the NodeJS delete() method.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?AWS=1499&DEVOPS=1999&Cloud=2499'); console.log("URL: ", Myurl.href); If we pass a key/value pair to the append() method, it will add them at the end of the query string. let params = new URLSearchParams('AWS=1499&DEVOPS=1999&Cloud=2499'); console.log("Query portion of the URL: " + params.toString()); //deletes 'DEVOPS' from the query string. params.delete('DEVOPS'); //The final Query string is: 'AWS=1499&Cloud=2499' console.log("After deleting 'DEVOPS': " + params.toString());
Output
As we can see in the output below, the name/value pairs which are having the name 'DEVOPS' are deleted.
URL: https://www.tutorialspoint.com/?AWS=1499&DEVOPS=1999&Cloud=2499 Query portion of the URL: AWS=1499&DEVOPS=1999&Cloud=2499 After deleting 'DEVOPS': AWS=1499&Cloud=2499
Example
The delete() method will remove all the name/value pairs from the query string which are having the name to be deleted.
In the following example, we are trying to delete the name/value pairs whose name is 'DEVOPS'.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999'); console.log("URL: ", Myurl.href); let params = new URLSearchParams('AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999'); console.log("Query portion of the URL: " + params.toString()); //deletes every pair with name 'DEVOPS' from the query string. params.delete('DEVOPS'); //The final Query string is: 'AWS=1499&Cloud=2499' console.log("After deleting 'DEVOPS': " + params.toString());
Output
As we can see in the output below, all the pair appearances of 'DEVOPS' are deleted from the query string.
URL: https://www.tutorialspoint.com/?AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999 Query portion of the URL: AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999 After deleting 'DEVOPS': AWS=1499&Cloud=2499
Example
The query string will remain the same if the name to be deleted is not in the input query string.
In the following example, we are trying to delete the name which is not in the query string.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999'); console.log("URL: ", Myurl.href); let params = new URLSearchParams('AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999'); console.log("Query portion of the URL: " + params.toString()); //deletes every pair with name 'GCP' from the query string. params.delete('GCP'); //The final Query string is: 'AWS=1499&Cloud=2499' console.log("After deleting 'GCP': " + params.toString());
Output
As we can see in the output, the input query string remains the same.
URL: https://www.tutorialspoint.com/?AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999 Query portion of the URL: AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999 After deleting 'GCP': AWS=1499&DEVOPS=1999&Cloud=2499&DEVOPS=4999&DEVOPS=5999
To Continue Learning Please Login